mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-21 21:20:07 -05:00
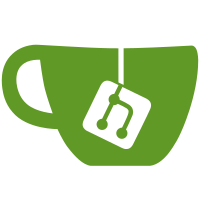
-Support 4k monitors, and in general, properly scale any monitor that is not HD. -Allow for a spatial filter of radius zero, which means do not use a spatial filter. -Add new variations: concentric, cpow3, helicoid, helix, rand_cubes, sphereblur. -Use a new method for computing elliptic which is more precise. Developed by Discord user Claude. -Remove the 8 variation per xform limitation on the GPU. -Allow for loading the last flame file on startup, rather than randoms. -Use two different default quality values in the interactive renderer, one each for CPU and GPU. -Creating linked xforms was using non-standard behavior. Make it match Apo and also support creating multiple linked xforms at once. --Bug fixes -No variations in an xform used to have the same behavior as a single linear variation with weight 1. While sensible, this breaks backward compatibility. No variations now sets the output point to zeroes. -Prevent crashing the program when adjusting a value on the main window while a final render is in progress. -The xaos table was inverted. --Code changes -Convert projects to Visual Studio 2017. -Change bad vals from +- 1e10 to +-1e20. -Reintroduce the symmetry tag in xforms for legacy support in programs that do not use color_speed. -Compiler will not let us use default values in templated member functions anymore.
413 lines
10 KiB
C++
413 lines
10 KiB
C++
#include "EmberCLPch.h"
|
|
#include "FunctionMapper.h"
|
|
|
|
namespace EmberCLns
|
|
{
|
|
std::unordered_map<string, string> FunctionMapper::s_GlobalMap;
|
|
|
|
FunctionMapper::FunctionMapper()
|
|
{
|
|
if (s_GlobalMap.empty())
|
|
{
|
|
s_GlobalMap["LRint"] =
|
|
"inline real_t LRint(real_t x)\n"
|
|
"{\n"
|
|
" intPrec temp = (x >= 0.0 ? (intPrec)(x + 0.5) : (intPrec)(x - 0.5));\n"
|
|
" return (real_t)temp;\n"
|
|
"}\n";
|
|
s_GlobalMap["Round"] =
|
|
"inline real_t Round(real_t r)\n"
|
|
"{\n"
|
|
" return (r > 0.0) ? floor(r + 0.5) : ceil(r - 0.5);\n"
|
|
"}\n";
|
|
s_GlobalMap["Sign"] =
|
|
"inline real_t Sign(real_t v)\n"
|
|
"{\n"
|
|
" return (v < 0.0) ? -1 : (v > 0.0) ? 1 : 0.0;\n"
|
|
"}\n";
|
|
s_GlobalMap["SignNz"] =
|
|
"inline real_t SignNz(real_t v)\n"
|
|
"{\n"
|
|
" return (v < 0.0) ? -1.0 : 1.0;\n"
|
|
"}\n";
|
|
s_GlobalMap["Sqr"] =
|
|
"inline real_t Sqr(real_t v)\n"
|
|
"{\n"
|
|
" return v * v;\n"
|
|
"}\n";
|
|
s_GlobalMap["SafeSqrt"] =
|
|
"inline real_t SafeSqrt(real_t x)\n"
|
|
"{\n"
|
|
" if (x <= 0.0)\n"
|
|
" return 0.0;\n"
|
|
"\n"
|
|
" return sqrt(x);\n"
|
|
"}\n";
|
|
s_GlobalMap["SafeDivInv"] =
|
|
"inline real_t SafeDivInv(real_t q, real_t r)\n"
|
|
"{\n"
|
|
" if (r < EPS)\n"
|
|
" return 1 / r;\n"
|
|
"\n"
|
|
" return q / r;\n"
|
|
"}\n";
|
|
s_GlobalMap["Cube"] =
|
|
"inline real_t Cube(real_t v)\n"
|
|
"{\n"
|
|
" return v * v * v;\n"
|
|
"}\n";
|
|
s_GlobalMap["Hypot"] =
|
|
"inline real_t Hypot(real_t x, real_t y)\n"
|
|
"{\n"
|
|
" return sqrt(SQR(x) + SQR(y));\n"
|
|
"}\n";
|
|
s_GlobalMap["Spread"] =
|
|
"inline real_t Spread(real_t x, real_t y)\n"
|
|
"{\n"
|
|
" return Hypot(x, y) * ((x) > 0.0 ? 1.0 : -1.0);\n"
|
|
"}\n";
|
|
s_GlobalMap["Powq4"] =
|
|
"inline real_t Powq4(real_t x, real_t y)\n"
|
|
"{\n"
|
|
" return pow(fabs(x), y) * SignNz(x);\n"
|
|
"}\n";
|
|
s_GlobalMap["Powq4c"] =
|
|
"inline real_t Powq4c(real_t x, real_t y)\n"
|
|
"{\n"
|
|
" return y == 1.0 ? x : Powq4(x, y);\n"
|
|
"}\n";
|
|
s_GlobalMap["Zeps"] =
|
|
"inline real_t Zeps(real_t x)\n"
|
|
"{\n"
|
|
" return x == 0.0 ? EPS : x;\n"
|
|
"}\n";
|
|
s_GlobalMap["Lerp"] =
|
|
"inline real_t Lerp(real_t a, real_t b, real_t p)\n"
|
|
"{\n"
|
|
" return a + (b - a) * p;\n"
|
|
"}\n";
|
|
s_GlobalMap["Fabsmod"] =
|
|
"inline real_t Fabsmod(real_t v)\n"
|
|
"{\n"
|
|
" real_t dummy;\n"
|
|
"\n"
|
|
" return modf(v, &dummy);\n"
|
|
"}\n";
|
|
s_GlobalMap["Fosc"] =
|
|
"inline real_t Fosc(real_t p, real_t amp, real_t ph)\n"
|
|
"{\n"
|
|
" return 0.5 - cos(p * amp + ph) * 0.5;\n"
|
|
"}\n";
|
|
s_GlobalMap["Foscn"] =
|
|
"inline real_t Foscn(real_t p, real_t ph)\n"
|
|
"{\n"
|
|
" return 0.5 - cos(p + ph) * 0.5;\n"
|
|
"}\n";
|
|
s_GlobalMap["LogScale"] =
|
|
"inline real_t LogScale(real_t x)\n"
|
|
"{\n"
|
|
" return x == 0.0 ? 0.0 : log((fabs(x) + 1) * M_E) * SignNz(x) / M_E;\n"
|
|
"}\n";
|
|
s_GlobalMap["LogMap"] =
|
|
"inline real_t LogMap(real_t x)\n"
|
|
"{\n"
|
|
" return x == 0.0 ? 0.0 : (M_E + log(x * M_E)) * 0.25 * SignNz(x);\n"
|
|
"}\n";
|
|
s_GlobalMap["ClampGte"] =
|
|
"inline real_t ClampGte(real_t val, real_t gte)\n"
|
|
"{\n"
|
|
" return (val < gte) ? gte : val;\n"
|
|
"}\n";
|
|
s_GlobalMap["Swap"] =
|
|
"inline void Swap(real_t* val1, real_t* val2)\n"
|
|
"{\n"
|
|
" real_t tmp = *val1;\n"
|
|
" *val1 = *val2;\n"
|
|
" *val2 = tmp;\n"
|
|
"}\n";
|
|
s_GlobalMap["Hash"] =
|
|
"inline real_t Hash(int a)\n"
|
|
"{\n"
|
|
" a = (a ^ 61) ^ (a >> 16);\n"
|
|
" a = a + (a << 3);\n"
|
|
" a = a ^ (a >> 4);\n"
|
|
" a = a * 0x27d4eb2d;\n"
|
|
" a = a ^ (a >> 15);\n"
|
|
" return (real_t)a / INT_MAX;\n"
|
|
"}\n";
|
|
s_GlobalMap["Vratio"] =
|
|
"inline real_t Vratio(real2* p, real2* q, real2* u)\n"
|
|
"{\n"
|
|
" real2 pmq = *p - *q;\n"
|
|
"\n"
|
|
" if (pmq.x == 0 && pmq.y == 0)\n"
|
|
" return 1.0;\n"
|
|
"\n"
|
|
" return 2 * (((*u).x - (*q).x) * pmq.x + ((*u).y - (*q).y) * pmq.y) / Zeps(SQR(pmq.x) + SQR(pmq.y));\n"
|
|
"}\n";
|
|
s_GlobalMap["Closest"] =
|
|
"inline int Closest(real2* p, int n, real2* u)\n"
|
|
"{\n"
|
|
" real_t d2;\n"
|
|
" real_t d2min = TMAX;\n"
|
|
" int i, j = 0;\n"
|
|
"\n"
|
|
" for (i = 0; i < n; i++)\n"
|
|
" {\n"
|
|
" d2 = Sqr(p[i].x - (*u).x) + Sqr(p[i].y - (*u).y);\n"
|
|
"\n"
|
|
" if (d2 < d2min)\n"
|
|
" {\n"
|
|
" d2min = d2;\n"
|
|
" j = i;\n"
|
|
" }\n"
|
|
" }\n"
|
|
"\n"
|
|
" return j;\n"
|
|
"}\n";
|
|
s_GlobalMap["Voronoi"] =
|
|
"inline real_t Voronoi(real2* p, int n, int q, real2* u)\n"
|
|
"{\n"
|
|
" real_t ratio;\n"
|
|
" real_t ratiomax = TLOW;\n"
|
|
" int i;\n"
|
|
"\n"
|
|
" for (i = 0; i < n; i++)\n"
|
|
" {\n"
|
|
" if (i != q)\n"
|
|
" {\n"
|
|
" ratio = Vratio(&p[i], &p[q], u);\n"
|
|
"\n"
|
|
" if (ratio > ratiomax)\n"
|
|
" ratiomax = ratio;\n"
|
|
" }\n"
|
|
" }\n"
|
|
"\n"
|
|
" return ratiomax;\n"
|
|
"}\n";
|
|
s_GlobalMap["SimplexNoise3D"] =
|
|
"inline real_t SimplexNoise3D(real4* v, __global real_t* p, __global real_t* grad)\n"
|
|
"{\n"
|
|
" real4 c[4];\n"
|
|
" real_t n = 0;\n"
|
|
" int gi[4];\n"
|
|
" real_t skewIn = ((*v).x + (*v).y + (*v).z) * 0.333333;\n"
|
|
" int i = (int)floor((*v).x + skewIn);\n"
|
|
" int j = (int)floor((*v).y + skewIn);\n"
|
|
" int k = (int)floor((*v).z + skewIn);\n"
|
|
" real_t t = (i + j + k) * 0.1666666;\n"
|
|
" real_t x0 = i - t;\n"
|
|
" real_t y0 = j - t;\n"
|
|
" real_t z0 = k - t;\n"
|
|
" c[0].x = (*v).x - x0;\n"
|
|
" c[0].y = (*v).y - y0;\n"
|
|
" c[0].z = (*v).z - z0;\n"
|
|
" int i1, j1, k1;\n"
|
|
" int i2, j2, k2;\n"
|
|
" real4 u;\n"
|
|
"\n"
|
|
" if (c[0].x >= c[0].y)\n"
|
|
" {\n"
|
|
" if (c[0].y >= c[0].z)\n"
|
|
" {\n"
|
|
" i1 = 1; j1 = 0; k1 = 0; i2 = 1; j2 = 1; k2 = 0;\n"
|
|
" }\n"
|
|
" else\n"
|
|
" {\n"
|
|
" if (c[0].x >= c[0].z)\n"
|
|
" {\n"
|
|
" i1 = 1; j1 = 0; k1 = 0; i2 = 1; j2 = 0; k2 = 1;\n"
|
|
" }\n"
|
|
" else\n"
|
|
" {\n"
|
|
" i1 = 0; j1 = 0; k1 = 1; i2 = 1; j2 = 0; k2 = 1;\n"
|
|
" }\n"
|
|
" }\n"
|
|
" }\n"
|
|
" else\n"
|
|
" {\n"
|
|
" if (c[0].y < c[0].z)\n"
|
|
" {\n"
|
|
" i1 = 0; j1 = 0; k1 = 1; i2 = 0; j2 = 1; k2 = 1;\n"
|
|
" }\n"
|
|
" else\n"
|
|
" {\n"
|
|
" if (c[0].x < c[0].z)\n"
|
|
" {\n"
|
|
" i1 = 0; j1 = 1; k1 = 0; i2 = 0; j2 = 1; k2 = 1;\n"
|
|
" }\n"
|
|
" else\n"
|
|
" {\n"
|
|
" i1 = 0; j1 = 1; k1 = 0; i2 = 1; j2 = 1; k2 = 0;\n"
|
|
" }\n"
|
|
" }\n"
|
|
" }\n"
|
|
"\n"
|
|
" c[1].x = c[0].x - i1 + 0.1666666;\n"
|
|
" c[1].y = c[0].y - j1 + 0.1666666;\n"
|
|
" c[1].z = c[0].z - k1 + 0.1666666;\n"
|
|
" c[2].x = c[0].x - i2 + 2 * 0.1666666;\n"
|
|
" c[2].y = c[0].y - j2 + 2 * 0.1666666;\n"
|
|
" c[2].z = c[0].z - k2 + 2 * 0.1666666;\n"
|
|
" c[3].x = c[0].x - 1 + 3 * 0.1666666;\n"
|
|
" c[3].y = c[0].y - 1 + 3 * 0.1666666;\n"
|
|
" c[3].z = c[0].z - 1 + 3 * 0.1666666;\n"
|
|
" int ii = i & 0x3ff;\n"
|
|
" int jj = j & 0x3ff;\n"
|
|
" int kk = k & 0x3ff;\n"
|
|
" gi[0] = (int)p[ii + (int)p[jj + (int)p[kk]]];\n"
|
|
" gi[1] = (int)p[ii + i1 + (int)p[jj + j1 + (int)p[kk + k1]]];\n"
|
|
" gi[2] = (int)p[ii + i2 + (int)p[jj + j2 + (int)p[kk + k2]]];\n"
|
|
" gi[3] = (int)p[ii + 1 + (int)p[jj + 1 + (int)p[kk + 1]]];\n"
|
|
"\n"
|
|
" for (uint corner = 0; corner < 4; corner++)\n"
|
|
" {\n"
|
|
" t = 0.6 - Sqr(c[corner].x) - Sqr(c[corner].y) - Sqr(c[corner].z);\n"
|
|
"\n"
|
|
" if (t > 0)\n"
|
|
" {\n"
|
|
" int index = gi[corner] * 3;\n"
|
|
" u.x = grad[index];\n"
|
|
" u.y = grad[index + 1];\n"
|
|
" u.z = grad[index + 2];\n"
|
|
" t *= t;\n"
|
|
" n += t * t * (u.x * c[corner].x + u.y * c[corner].y + u.z * c[corner].z);\n"
|
|
" }\n"
|
|
" }\n"
|
|
"\n"
|
|
" return 32.0 * n;\n"
|
|
"}\n";
|
|
s_GlobalMap["PerlinNoise3D"] =
|
|
"inline real_t PerlinNoise3D(real4* v, __global real_t* p, __global real_t* grad, real_t aScale, real_t fScale, int octaves)\n"
|
|
"{\n"
|
|
" int i;\n"
|
|
" real_t n = 0.0, a = 1.0;\n"
|
|
" real4 u = *v;\n"
|
|
"\n"
|
|
" for (i = 0; i < octaves; i++)\n"
|
|
" {\n"
|
|
" n += SimplexNoise3D(&u, p, grad) / Zeps(a);\n"
|
|
" a *= aScale;\n"
|
|
" u.x *= fScale;\n"
|
|
" u.y *= fScale;\n"
|
|
" u.x *= fScale;\n"
|
|
" }\n"
|
|
"\n"
|
|
" return n;\n"
|
|
"}\n";
|
|
s_GlobalMap["JacobiElliptic"] =
|
|
"inline void JacobiElliptic(real_t uu, real_t emmc, real_t* sn, real_t* cn, real_t* dn)\n"
|
|
"{\n"
|
|
" real_t CA = 0.0003;\n"
|
|
" real_t a, b, c, d = 1, em[13], en[13];\n"
|
|
" int bo;\n"
|
|
" int l;\n"
|
|
" int ii;\n"
|
|
" int i;\n"
|
|
" real_t emc = emmc;\n"
|
|
" real_t u = uu;\n"
|
|
"\n"
|
|
" if (emc != 0)\n"
|
|
" {\n"
|
|
" bo = 0;\n"
|
|
"\n"
|
|
" if (emc < 0)\n"
|
|
" bo = 1;\n"
|
|
"\n"
|
|
" if (bo != 0)\n"
|
|
" {\n"
|
|
" d = 1 - emc;\n"
|
|
" emc = -emc / d;\n"
|
|
" d = sqrt(d);\n"
|
|
" u = d * u;\n"
|
|
" }\n"
|
|
"\n"
|
|
" a = 1;\n"
|
|
" *dn = 1;\n"
|
|
"\n"
|
|
" for (i = 0; i < 8; i++)\n"
|
|
" {\n"
|
|
" l = i;\n"
|
|
" em[i] = a;\n"
|
|
" emc = sqrt(emc);\n"
|
|
" en[i] = emc;\n"
|
|
" c = 0.5 * (a + emc);\n"
|
|
"\n"
|
|
" if (fabs(a - emc) <= CA * a)\n"
|
|
" break;\n"
|
|
"\n"
|
|
" emc = a * emc;\n"
|
|
" a = c;\n"
|
|
" }\n"
|
|
"\n"
|
|
" u = c * u;\n"
|
|
" *sn = sincos(u, cn);\n"
|
|
"\n"
|
|
" if (*sn != 0)\n"
|
|
" {\n"
|
|
" a = *cn / *sn;\n"
|
|
" c = a * c;\n"
|
|
"\n"
|
|
" for (ii = l; ii >= 0; --ii)\n"
|
|
" {\n"
|
|
" b = em[ii];\n"
|
|
" a = c * a;\n"
|
|
" c = *dn * c;\n"
|
|
" *dn = (en[ii] + a) / (b + a);\n"
|
|
" a = c / b;\n"
|
|
" }\n"
|
|
"\n"
|
|
" a = 1 / sqrt(c * c + 1);\n"
|
|
"\n"
|
|
" if (*sn < 0)\n"
|
|
" *sn = -a;\n"
|
|
" else\n"
|
|
" *sn = a;\n"
|
|
"\n"
|
|
" *cn = c * *sn;\n"
|
|
" }\n"
|
|
"\n"
|
|
" if (bo != 0)\n"
|
|
" {\n"
|
|
" a = *dn;\n"
|
|
" *dn = *cn;\n"
|
|
" *cn = a;\n"
|
|
" *sn = *sn / d;\n"
|
|
" }\n"
|
|
" }\n"
|
|
" else\n"
|
|
" {\n"
|
|
" *cn = 1 / cosh(u);\n"
|
|
" *dn = *cn;\n"
|
|
" *sn = tanh(u);\n"
|
|
" }\n"
|
|
"}\n";
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Get a pointer to the text of the global function whose name is the passed in string.
|
|
/// </summary>
|
|
/// <param name="func">The function name to retrieve</param>
|
|
/// <returns>A pointer to the function body string if found, else nullptr.</returns>
|
|
const string* FunctionMapper::GetGlobalFunc(const string& func)
|
|
{
|
|
const auto& text = s_GlobalMap.find(func);
|
|
|
|
if (text != s_GlobalMap.end())
|
|
return &text->second;
|
|
else
|
|
return nullptr;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Get a copy of the function map.
|
|
/// This is useful only for debugging/testing.
|
|
/// </summary>
|
|
/// <returns>A copy of the function map</returns>
|
|
const std::unordered_map<string, string> FunctionMapper::GetGlobalMapCopy()
|
|
{
|
|
return s_GlobalMap;
|
|
}
|
|
}
|