mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-11 18:54:53 -04:00
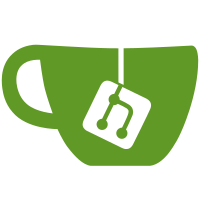
-Add backward compatibility option for the following variations: cos, cosh, cot, coth, csc, csch, sec, sech, sin, sinh, tan, tanh. -Add the ability to re-order variations by dragging them in the Info tab.
55 lines
1.2 KiB
C++
55 lines
1.2 KiB
C++
#pragma once
|
|
|
|
#include "FractoriumPch.h"
|
|
|
|
class Fractorium;
|
|
|
|
/// <summary>
|
|
/// A thin derivation of QTreeWidget which allows for processing the drop event.
|
|
/// </summary>
|
|
class LibraryTreeWidget : public QTreeWidget
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
/// <summary>
|
|
/// Constructor that passes p to the parent.
|
|
/// </summary>
|
|
/// <param name="p">The parent widget</param>
|
|
explicit LibraryTreeWidget(QWidget* p = nullptr)
|
|
: QTreeWidget(p)
|
|
{
|
|
}
|
|
|
|
void SetMainWindow(Fractorium* f);
|
|
|
|
protected:
|
|
virtual void dropEvent(QDropEvent* de) override;
|
|
|
|
Fractorium* m_Fractorium = nullptr;
|
|
};
|
|
|
|
|
|
class InfoTreeWidget : public QTreeWidget
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
/// <summary>
|
|
/// Constructor that passes p to the parent.
|
|
/// </summary>
|
|
/// <param name="p">The parent widget</param>
|
|
explicit InfoTreeWidget(QWidget* p = nullptr)
|
|
: QTreeWidget(p)
|
|
{
|
|
}
|
|
|
|
void SetMainWindow(Fractorium* f);
|
|
const QString& LastNonVarField() const { return m_LastNonVarField; }
|
|
|
|
protected:
|
|
virtual void dropEvent(QDropEvent* de) override;
|
|
virtual void dragMoveEvent(QDragMoveEvent* dme) override;
|
|
|
|
Fractorium* m_Fractorium = nullptr;
|
|
QString m_LastNonVarField = "Direct color";//It is critical to update this if any more fields are ever added before the variations start.
|
|
};
|