mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-03 14:54:52 -04:00
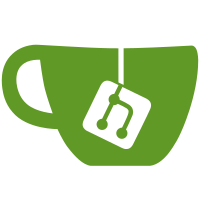
-Add a new dialog for editing QSS stylesheets. Allow for saving, reloading and setting styles as default. --Include a dark style with the installation called dark.qss. --Also add support for themes such as Fusion. --Resize some controls to better fit with the new style. -Add an option to specify the number of random embers generated on startup. 1 is the minimum and the default. --Bug fixes -Properly enable/disable thread priority label in final render dialog in response to enable/disable of the OpenCL checkbox. -Remove all inline stylesheets. -Show xaos spinners with 6 decimal places. --Code changes -Remove redundant comparisons to nullptr, use ! instead; -Give some controls valid names instead of the auto generated ones. -DoubleSpinBoxTableItemDelegate.h: Add virtual keyword to overridden functions.
121 lines
3.1 KiB
C++
121 lines
3.1 KiB
C++
#include "FractoriumPch.h"
|
|
#include "Fractorium.h"
|
|
#include "QssDialog.h"
|
|
|
|
/// <summary>
|
|
/// Initialize the toolbar UI.
|
|
/// </summary>
|
|
void Fractorium::InitToolbarUI()
|
|
{
|
|
auto clGroup = new QActionGroup(this);
|
|
clGroup->addAction(ui.ActionCpu);
|
|
clGroup->addAction(ui.ActionCL);
|
|
|
|
auto spGroup = new QActionGroup(this);
|
|
spGroup->addAction(ui.ActionSP);
|
|
spGroup->addAction(ui.ActionDP);
|
|
|
|
SyncOptionsToToolbar();
|
|
connect(ui.ActionCpu, SIGNAL(triggered(bool)), this, SLOT(OnActionCpu(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionCL, SIGNAL(triggered(bool)), this, SLOT(OnActionCL(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionSP, SIGNAL(triggered(bool)), this, SLOT(OnActionSP(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionDP, SIGNAL(triggered(bool)), this, SLOT(OnActionDP(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionStyle, SIGNAL(triggered(bool)), this, SLOT(OnActionStyle(bool)), Qt::QueuedConnection);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the CPU render option on the toolbar is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, action only taken if true.</param>
|
|
void Fractorium::OnActionCpu(bool checked)
|
|
{
|
|
if (checked && m_Settings->OpenCL())
|
|
{
|
|
m_Settings->OpenCL(false);
|
|
ShutdownAndRecreateFromOptions();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the OpenCL render option on the toolbar is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, action only taken if true.</param>
|
|
void Fractorium::OnActionCL(bool checked)
|
|
{
|
|
if (checked && !m_Settings->OpenCL())
|
|
{
|
|
m_Settings->OpenCL(true);
|
|
ShutdownAndRecreateFromOptions();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the single precision render option on the toolbar is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, action only taken if true.</param>
|
|
void Fractorium::OnActionSP(bool checked)
|
|
{
|
|
if (checked && m_Settings->Double())
|
|
{
|
|
m_Settings->Double(false);
|
|
ShutdownAndRecreateFromOptions();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the double precision render option on the toolbar is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, action only taken if true.</param>
|
|
void Fractorium::OnActionDP(bool checked)
|
|
{
|
|
if (checked && !m_Settings->Double())
|
|
{
|
|
m_Settings->Double(true);
|
|
ShutdownAndRecreateFromOptions();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the show style button is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Ignored</param>
|
|
void Fractorium::OnActionStyle(bool checked)
|
|
{
|
|
m_QssDialog->show();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Sync options data to the check state of the toolbar buttons.
|
|
/// This does not trigger a clicked() event.
|
|
/// </summary>
|
|
void Fractorium::SyncOptionsToToolbar()
|
|
{
|
|
static bool openCL = !OpenCLInfo::Instance().Devices().empty();
|
|
|
|
if (!openCL)
|
|
{
|
|
ui.ActionCL->setEnabled(false);
|
|
}
|
|
|
|
if (openCL && m_Settings->OpenCL())
|
|
{
|
|
ui.ActionCpu->setChecked(false);
|
|
ui.ActionCL->setChecked(true);
|
|
}
|
|
else
|
|
{
|
|
ui.ActionCpu->setChecked(true);
|
|
ui.ActionCL->setChecked(false);
|
|
}
|
|
|
|
if (m_Settings->Double())
|
|
{
|
|
ui.ActionSP->setChecked(false);
|
|
ui.ActionDP->setChecked(true);
|
|
}
|
|
else
|
|
{
|
|
ui.ActionSP->setChecked(true);
|
|
ui.ActionDP->setChecked(false);
|
|
}
|
|
} |