mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-12 03:04:51 -04:00
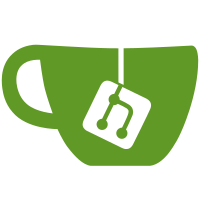
Add the ability to apply operations to a selection of xforms, rather than just the current one. Update tool tips accordingly. --Bug fixes Fix NAN in equalized weight calculation when all weights are set to 0. --Code changes Clean up strange nested usage of Update([&]()) calls when changing xform color index via either the spinner or scroll bar. Made no sense. Make ISAAC RandBit() use RandByte() to be slightly more efficient. Put FillXforms() in the controller where it belongs, rather than the main window class. Add UpdateXform() function to the controller to handle applying operations to multiple xforms. Remove the word "Current" out of most xform related functions because they now operate on whatever is selected. Properly use Update() for various xform operations whereas before it was manually calling UpdateRender(). Also properly use Update() in places where it was erroneously using UpdateXform() for things that did not involve xforms. Block signals in FillXaosTable(). Add new file named FractoriumXformsSelect.cpp to handle new xform selection code.
128 lines
4.0 KiB
C++
128 lines
4.0 KiB
C++
#include "FractoriumPch.h"
|
|
#include "Fractorium.h"
|
|
|
|
/// <summary>
|
|
/// Initialize the xforms selection UI.
|
|
/// </summary>
|
|
void Fractorium::InitXformsSelectUI()
|
|
{
|
|
m_XformsSelectionLayout = (QFormLayout*)ui.XformsSelectGroupBoxScrollAreaWidget->layout();
|
|
connect(ui.XformsSelectAllButton, SIGNAL(clicked(bool)), this, SLOT(OnXformsSelectAllButtonClicked(bool)), Qt::QueuedConnection);
|
|
connect(ui.XformsSelectNoneButton, SIGNAL(clicked(bool)), this, SLOT(OnXformsSelectNoneButtonClicked(bool)), Qt::QueuedConnection);
|
|
|
|
ClearXformsSelections();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Check all of the xform selection checkboxes.
|
|
/// </summary>
|
|
/// <param name="checked">Ignored</param>
|
|
void Fractorium::OnXformsSelectAllButtonClicked(bool checked) { ForEachXformCheckbox([&](int i, QCheckBox* w) { w->setChecked(true); }); }
|
|
|
|
/// <summary>
|
|
/// Uncheck all of the xform selection checkboxes.
|
|
/// </summary>
|
|
/// <param name="checked">Ignored</param>
|
|
void Fractorium::OnXformsSelectNoneButtonClicked(bool checked) { ForEachXformCheckbox([&](int i, QCheckBox* w) { w->setChecked(false); }); }
|
|
|
|
/// <summary>
|
|
/// Clear all of the dynamically created xform checkboxes.
|
|
/// </summary>
|
|
void Fractorium::ClearXformsSelections()
|
|
{
|
|
QLayoutItem* child = nullptr;
|
|
|
|
m_XformSelections.clear();
|
|
m_XformsSelectionLayout->blockSignals(true);
|
|
|
|
while (m_XformsSelectionLayout->count() && (child = m_XformsSelectionLayout->takeAt(0)))
|
|
{
|
|
auto* w = child->widget();
|
|
delete child;
|
|
delete w;
|
|
}
|
|
|
|
m_XformsSelectionLayout->blockSignals(false);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Make a caption from an xform.
|
|
/// The caption will be the xform count + 1, optionally followed by the xform's name.
|
|
/// For final xforms, the string "Final" will be used in place of the count.
|
|
/// </summary>
|
|
/// <param name="i">The index of xform to make a caption for</param>
|
|
/// <returns>The caption string</returns>
|
|
template <typename T>
|
|
QString FractoriumEmberController<T>::MakeXformCaption(size_t i)
|
|
{
|
|
bool isFinal = m_Ember.FinalXform() == m_Ember.GetTotalXform(i);
|
|
QString caption = isFinal ? "Final" : QString::number(i + 1);
|
|
|
|
if (Xform<T>* xform = m_Ember.GetTotalXform(i))
|
|
{
|
|
if (!xform->m_Name.empty())
|
|
caption += " (" + QString::fromStdString(xform->m_Name) + ")";
|
|
}
|
|
|
|
return caption;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Function to perform the specified operation on every dynamically created xform selection checkbox.
|
|
/// </summary>
|
|
/// <param name="func">The operation to perform</param>
|
|
void Fractorium::ForEachXformCheckbox(std::function<void(int, QCheckBox*)> func)
|
|
{
|
|
int i = 0;
|
|
|
|
while (QLayoutItem* child = m_XformsSelectionLayout->itemAt(i))
|
|
{
|
|
if (auto* w = dynamic_cast<QCheckBox*>(child->widget()))
|
|
{
|
|
func(i, w);
|
|
}
|
|
|
|
i++;
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Function to perform the specified operation on one dynamically created xform selection checkbox.
|
|
/// </summary>
|
|
/// <param name="i">The index of the checkbox</param>
|
|
/// <param name="func">The operation to perform</param>
|
|
/// <returns>True if the checkbox was found, else false.</returns>
|
|
template <typename T>
|
|
bool FractoriumEmberController<T>::XformCheckboxAt(int i, std::function<void(QCheckBox*)> func)
|
|
{
|
|
if (QLayoutItem* child = m_Fractorium->m_XformsSelectionLayout->itemAt(i))
|
|
{
|
|
if (auto* w = dynamic_cast<QCheckBox*>(child->widget()))
|
|
{
|
|
func(w);
|
|
return true;
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Function to perform the specified operation on one dynamically created xform selection checkbox.
|
|
/// The checkbox is specified by the xform it corresponds to, rather than its index.
|
|
/// </summary>
|
|
/// <param name="xform">The xform that corresponds to the checkbox</param>
|
|
/// <param name="func">The operation to perform</param>
|
|
/// <returns>True if the checkbox was found, else false.</returns>
|
|
template <typename T>
|
|
bool FractoriumEmberController<T>::XformCheckboxAt(Xform<T>* xform, std::function<void(QCheckBox*)> func)
|
|
{
|
|
return XformCheckboxAt(m_Ember.GetTotalXformIndex(xform), func);
|
|
}
|
|
|
|
template class FractoriumEmberController<float>;
|
|
|
|
#ifdef DO_DOUBLE
|
|
template class FractoriumEmberController<double>;
|
|
#endif
|