mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-05 15:54:50 -04:00
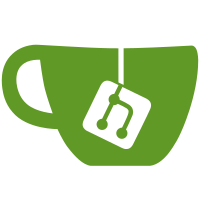
-Remove the option --intpalette to format the palette in the xml as ints. If they are not hex formatted, then they should always be float. This option was pointless. -Cleanup some options text for the command line programs. -Allow for dragging around flames in the library tab. This is useful for setting up the order of an animation. -Make the opening of large files in Fractorium much more efficient when not-appending. -Make the opening of large files in all EmberRender and EmberAnimate more efficient. -Better error reporting when opening files. --Bug fixes -Get rid of leftover artifacts that would appear on preview thumbnails when either switching SP/DP or re-rendering previews. -Filename extension was not being appended on Linux when saving as Xml, thus making it impossible to drag that file back in becase drop is filtered on extension. --Code changes -Move GCC compiler spec to C++14. Building with 5.3 now on linux. -Use inline member data initializers. -Make a #define for static for use in Utils.h to make things a little cleaner. -Make various functions able to take arbitrary collections as their parameters rather than just vectors. -Make library collection a list rather than vector. This alleviates the need to re-sync pointers whenever the collection changes. -Subclass QTreeWidget for the library tree. Two new files added for this. -Remove all usage of #ifdef ROW_ONLY_DE in DEOpenCLKernelCreator, it was never used. -Add move constructor and assignment operator to EmberFile. -Add the ability to use a pointer to outside memory in the renderer for the vector of Ember<T>. -Make a lot more functions const where they should be.
64 lines
1.5 KiB
C++
64 lines
1.5 KiB
C++
#pragma once
|
|
|
|
#include "ui_OptionsDialog.h"
|
|
#include "FractoriumSettings.h"
|
|
#include "SpinBox.h"
|
|
|
|
/// <summary>
|
|
/// FractoriumOptionsDialog class.
|
|
/// </summary>
|
|
|
|
class Fractorium;//Forward declaration since Fractorium uses this dialog.
|
|
|
|
/// <summary>
|
|
/// The options dialog allows the user to save various preferences
|
|
/// between program runs.
|
|
/// It has a pointer to a FractoriumSettings object which is assigned
|
|
/// in the constructor. The main window holds the object as a member and the
|
|
/// pointer to it here is just for convenience.
|
|
/// </summary>
|
|
class FractoriumOptionsDialog : public QDialog
|
|
{
|
|
Q_OBJECT
|
|
|
|
friend Fractorium;
|
|
|
|
public:
|
|
FractoriumOptionsDialog(FractoriumSettings* settings, QWidget* p = nullptr, Qt::WindowFlags f = 0);
|
|
|
|
public slots:
|
|
void OnOpenCLCheckBoxStateChanged(int state);
|
|
void OnDeviceTableCellChanged(int row, int col);
|
|
void OnDeviceTableRadioToggled(bool checked);
|
|
virtual void accept() override;
|
|
virtual void reject() override;
|
|
|
|
protected:
|
|
virtual void showEvent(QShowEvent* e) override;
|
|
|
|
private:
|
|
bool EarlyClip();
|
|
bool YAxisUp();
|
|
bool AlphaChannel();
|
|
bool Transparency();
|
|
bool ContinuousUpdate();
|
|
bool OpenCL();
|
|
bool Double();
|
|
bool ShowAllXforms();
|
|
bool AutoUnique();
|
|
uint ThreadCount();
|
|
uint RandomCount();
|
|
void DataToGui();
|
|
void GuiToData();
|
|
|
|
Ui::OptionsDialog ui;
|
|
shared_ptr<OpenCLInfo> m_Info;
|
|
SpinBox* m_XmlTemporalSamplesSpin;
|
|
SpinBox* m_XmlQualitySpin;
|
|
SpinBox* m_XmlSupersampleSpin;
|
|
QLineEdit* m_IdEdit;
|
|
QLineEdit* m_UrlEdit;
|
|
QLineEdit* m_NickEdit;
|
|
FractoriumSettings* m_Settings;
|
|
};
|