mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-22 13:40:06 -05:00
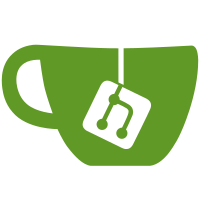
-Support 4k monitors, and in general, properly scale any monitor that is not HD. -Allow for a spatial filter of radius zero, which means do not use a spatial filter. -Add new variations: concentric, cpow3, helicoid, helix, rand_cubes, sphereblur. -Use a new method for computing elliptic which is more precise. Developed by Discord user Claude. -Remove the 8 variation per xform limitation on the GPU. -Allow for loading the last flame file on startup, rather than randoms. -Use two different default quality values in the interactive renderer, one each for CPU and GPU. -Creating linked xforms was using non-standard behavior. Make it match Apo and also support creating multiple linked xforms at once. --Bug fixes -No variations in an xform used to have the same behavior as a single linear variation with weight 1. While sensible, this breaks backward compatibility. No variations now sets the output point to zeroes. -Prevent crashing the program when adjusting a value on the main window while a final render is in progress. -The xaos table was inverted. --Code changes -Convert projects to Visual Studio 2017. -Change bad vals from +- 1e10 to +-1e20. -Reintroduce the symmetry tag in xforms for legacy support in programs that do not use color_speed. -Compiler will not let us use default values in templated member functions anymore.
77 lines
2.8 KiB
C++
77 lines
2.8 KiB
C++
#pragma once
|
|
|
|
#include "Utils.h"
|
|
#include "PaletteList.h"
|
|
#include "VariationList.h"
|
|
#include "Ember.h"
|
|
|
|
#ifdef __APPLE__
|
|
#include <libgen.h>
|
|
#endif
|
|
|
|
/// <summary>
|
|
/// XmlToEmber and Locale classes.
|
|
/// </summary>
|
|
|
|
namespace EmberNs
|
|
{
|
|
/// <summary>
|
|
/// Convenience class for setting and resetting the locale.
|
|
/// It's set up in the constructor and restored in the destructor.
|
|
/// This relieves the caller of having to manually do it everywhere.
|
|
/// </summary>
|
|
class EMBER_API Locale
|
|
{
|
|
public:
|
|
Locale(int category = LC_NUMERIC, const char* loc = "C");
|
|
~Locale();
|
|
|
|
private:
|
|
int m_Category;
|
|
string m_NewLocale;
|
|
string m_OriginalLocale;
|
|
};
|
|
|
|
/// <summary>
|
|
/// Class for reading Xml files into ember objects.
|
|
/// This class derives from EmberReport, so the caller is able
|
|
/// to retrieve a text dump of error information if any errors occur.
|
|
/// Since this class contains a VariationList object, it's important to declare one
|
|
/// instance and reuse it for the duration of the program instead of creating and deleting
|
|
/// them as local variables.
|
|
/// Template argument expected to be float or double.
|
|
/// </summary>
|
|
template <typename T>
|
|
class EMBER_API XmlToEmber : public EmberReport
|
|
{
|
|
public:
|
|
XmlToEmber();
|
|
template <typename Alloc, template <typename, typename> class C>
|
|
bool Parse(byte* buf, const char* filename, C<Ember<T>, Alloc>& embers, bool useDefaults);
|
|
template <typename Alloc, template <typename, typename> class C>
|
|
bool Parse(const char* filename, C<Ember<T>, Alloc>& embers, bool useDefaults);
|
|
template <typename valT>
|
|
bool Aton(const char* str, valT& val);
|
|
static vector<string> m_FlattenNames;
|
|
|
|
private:
|
|
template <typename Alloc, template <typename, typename> class C>
|
|
void ScanForEmberNodes(xmlNode* curNode, const char* parentFile, C<Ember<T>, Alloc>& embers, bool useDefaults);
|
|
bool ParseEmberElement(xmlNode* emberNode, Ember<T>& currentEmber);
|
|
bool AttToEmberMotionFloat(xmlAttrPtr att, const char* attStr, eEmberMotionParam param, EmberMotion<T>& motion);
|
|
bool ParseXform(xmlNode* childNode, Xform<T>& xform, bool motion, bool fromEmber);
|
|
static string GetCorrectedParamName(const unordered_map<string, string>& names, const char* name);
|
|
static string GetCorrectedVariationName(vector<pair<pair<string, string>, vector<string>>>& vec, xmlAttrPtr att);
|
|
static bool XmlContainsTag(xmlAttrPtr att, const char* name);
|
|
bool ParseHexColors(const char* colstr, Ember<T>& ember, size_t numColors, intmax_t chan);
|
|
template <typename valT>
|
|
bool ParseAndAssign(const xmlChar* name, const char* attStr, const char* str, valT& val, bool& b);
|
|
|
|
static bool m_Init;
|
|
static unordered_map<string, string> m_BadParamNames;
|
|
static vector<pair<pair<string, string>, vector<string>>> m_BadVariationNames;
|
|
shared_ptr<VariationList<T>> m_VariationList;//The variation list used to make copies of variations to populate the embers with.
|
|
shared_ptr<PaletteList<float>> m_PaletteList;
|
|
};
|
|
}
|