mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-18 14:14:53 -04:00
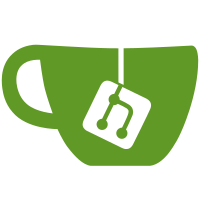
-Add a palette editor. -Add support for reading .ugr/.gradient/.gradients palette files. -Allow toggling on spinners whose minimum value is not zero. -Allow toggling display of image, affines and grid. -Add new variations: cylinder2, circlesplit, tile_log, truchet_fill, waves2_radial. --Bug fixes -cpow2 was wrong. -Palettes with rapid changes in color would produce slightly different outputs from Apo/Chaotica. This was due to a long standing bug from flam3. -Use exec() on Apple and show() on all other OSes for dialog boxes. -Trying to render a sequence with no frames would crash. -Selecting multiple xforms and rotating them would produce the wrong rotation. -Better handling when parsing flames using different encoding, such as unicode and UTF-8. -Switching between SP/DP didn't reselect the selected flame in the Library tab. --Code changes -Make all types concerning palettes be floats, including PaletteTableWidgetItem. -PaletteTableWidgetItem is no longer templated because all palettes are float. -Include the source colors for user created gradients. -Change parallel_for() calls to work with very old versions of TBB which are lingering on some systems. -Split conditional out of accumulation loop on the CPU for better performance. -Vectorize summing when doing density filter for better performance. -Make all usage of palettes be of type float, double is pointless. -Allow palettes to reside in multiple folders, while ensuring only one of each name is added. -Refactor some palette path searching code. -Make ReadFile() throw and catch an exception if the file operation fails. -A little extra safety in foci and foci3D with a call to Zeps(). -Cast to (real_t) in the OpenCL string for the w variation, which was having trouble compiling on Mac. -Fixing missing comma between paths in InitPaletteList(). -Move Xml and PaletteList classes into cpp to shorten build times when working on them. -Remove default param values for IterOpenCLKernelCreator<T>::SharedDataIndexDefines in cpp file. -Change more NULL to nullptr.
139 lines
2.8 KiB
C++
139 lines
2.8 KiB
C++
#ifndef FRACTORIUM_PCH_H
|
|
#define FRACTORIUM_PCH_H//GCC doesn't like #pragma once
|
|
|
|
#define XFORM_COLOR_COUNT 14
|
|
|
|
#ifdef _WIN32
|
|
#pragma warning(disable : 4251; disable : 4661; disable : 4100)
|
|
#endif
|
|
|
|
//Has to come first on non-Windows platforms due to some weird naming collisions on *nix.
|
|
#ifndef _WIN32
|
|
#include <QtWidgets>
|
|
#endif
|
|
|
|
#include "Renderer.h"
|
|
#include "RendererCL.h"
|
|
#include "VariationList.h"
|
|
#include "OpenCLWrapper.h"
|
|
#include "XmlToEmber.h"
|
|
#include "EmberToXml.h"
|
|
#include "SheepTools.h"
|
|
#include "JpegUtils.h"
|
|
#include "EmberCommon.h"
|
|
|
|
#ifdef _WIN32
|
|
#include <QtWidgets>
|
|
#endif
|
|
|
|
#include <math.h>
|
|
#include <deque>
|
|
#include "qfunctions.h"
|
|
|
|
#include <QApplication>
|
|
#include <QBrush>
|
|
#include <QCheckBox>
|
|
#include <QClipboard>
|
|
#include <QColor>
|
|
#include <QColorDialog>
|
|
#include <QComboBox>
|
|
#include <QConicalGradient>
|
|
#include <QDebug>
|
|
#include <QDesktopWidget>
|
|
#include <QDial>
|
|
#include <QDoubleSpinBox>
|
|
#include <QEvent>
|
|
#include <QFile>
|
|
#include <QFileInfo>
|
|
#include <QFont>
|
|
#include <QFontDialog>
|
|
#include <QFontMetrics>
|
|
#include <QFrame>
|
|
#include <QFuture>
|
|
#include <QGraphicsView>
|
|
#include <QGridLayout>
|
|
#include <QGroupBox>
|
|
#include <QHash>
|
|
#include <QHBoxLayout>
|
|
#include <QIcon>
|
|
#include <QImage>
|
|
#include <QImageReader>
|
|
#include <QItemDelegate>
|
|
#include <QKeyEvent>
|
|
#include <QLabel>
|
|
#include <QLayout>
|
|
#include <QLinearGradient>
|
|
#include <QLineEdit>
|
|
#include <QMap>
|
|
#include <QMenu>
|
|
#include <QMessageBox>
|
|
#include <QMimeData>
|
|
#include <QModelIndex>
|
|
#include <QMouseEvent>
|
|
#include <qopenglfunctions_2_0.h>
|
|
#include <QOpenGLWidget>
|
|
#include <QPainter>
|
|
#include <QPainterPath>
|
|
#include <QPaintEvent>
|
|
#include <QPixmap>
|
|
#include <QPoint>
|
|
#include <QPolygon>
|
|
#include <QPushButton>
|
|
#include <QRect>
|
|
#include <QResizeEvent>
|
|
#include <QSettings>
|
|
#include <QSignalMapper>
|
|
#include <QSize>
|
|
#include <QSizePolicy>
|
|
#include <QSpinBox>
|
|
#include <QStandardPaths>
|
|
#include <QtConcurrentRun>
|
|
#include <QtCore/qglobal.h>
|
|
#include <QtCore/QMultiHash>
|
|
#include <QtCore/QPair>
|
|
#include <QtCore/QSharedData>
|
|
#include <QtCore/QSize>
|
|
#include <QtCore/QStringList>
|
|
#include <QtCore/QVariant>
|
|
#include <QtCore/QVector>
|
|
#include <QTextEdit>
|
|
#include <QTextStream>
|
|
#include <QtGui/QFont>
|
|
#include <QtGui/QPalette>
|
|
#include <QtGui/QSyntaxHighlighter>
|
|
#include <QThread>
|
|
#include <QTime>
|
|
#include <QTimer>
|
|
#include <QToolBar>
|
|
#include <QToolTip>
|
|
#include <QTreeWidget>
|
|
#include <QtWidgets/QMainWindow>
|
|
#include <QVarLengthArray>
|
|
#include <QVBoxLayout>
|
|
#include <QVector>
|
|
#include <QWheelEvent>
|
|
#include <QWidget>
|
|
#include <QWidgetAction>
|
|
|
|
#define GLM_FORCE_RADIANS 1
|
|
#define GLM_ENABLE_EXPERIMENTAL 1
|
|
|
|
#ifndef __APPLE__
|
|
#define GLM_FORCE_INLINE 1
|
|
#endif
|
|
|
|
#include "glm/glm.hpp"
|
|
#include "glm/gtc/matrix_transform.hpp"
|
|
#include "glm/gtc/type_ptr.hpp"
|
|
|
|
#ifndef _WIN32
|
|
#undef Bool
|
|
#endif
|
|
|
|
using namespace std;
|
|
using namespace EmberNs;
|
|
using namespace EmberCLns;
|
|
using namespace EmberCommon;
|
|
|
|
#endif
|