mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-22 13:40:06 -05:00
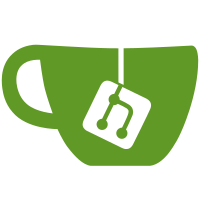
-Add new variations: crackle, dc_perlin. -Make default palette interp mode be linear instead of step. -Make summary tab the selected one in the Info tab. -Allow for highlight power of up to 10. It was previously limited to 2. --Bug fixes -Direct color calculations were wrong. -Flattening was not applied to final xform. -Fix "pure virtual function call" error on shutdown. --Code changes -Allow for array precalc params in variations by adding a size member to the ParamWithName class. -In IterOpenCLKernelCreator, memcpy precalc params instead of a direct assign since they can now be of variable length. -Add new file VarFuncs to consolidate some functions that are common to multiple variations. This also contains texture data for crackle and dc_perlin. -Place OpenCL versions of these functions in the FunctionMapper class in the EmberCL project. -Add new Singleton class that uses CRTP, is thread safe, and deletes after the last reference goes away. This fixes the usual "delete after main()" problem with singletons that use the static local function variable pattern. -Began saving files with AStyle autoformatter turned on. This will eventually touch all files as they are worked on. -Add missing backslash to CUDA include and library paths for builds on Nvidia systems. -Add missing gl.h include for Windows. -Remove glew include paths from Fractorium, it's not used. -Remove any Nvidia specific #defines and build targets, they are no longer needed with OpenCL 1.2. -Fix bad paths on linux build. -General cleanup.
136 lines
4.4 KiB
C++
136 lines
4.4 KiB
C++
#pragma once
|
|
|
|
#include "ui_FinalRenderDialog.h"
|
|
#include "SpinBox.h"
|
|
#include "DoubleSpinBox.h"
|
|
#include "TwoButtonComboWidget.h"
|
|
#include "FractoriumSettings.h"
|
|
#include "FinalRenderEmberController.h"
|
|
|
|
/// <summary>
|
|
/// FractoriumFinalRenderDialog class.
|
|
/// </summary>
|
|
|
|
class Fractorium;//Forward declaration since Fractorium uses this dialog.
|
|
class FinalRenderEmberControllerBase;
|
|
template <typename T> class FinalRenderEmberController;
|
|
|
|
/// <summary>
|
|
/// The final render dialog is for when the user is satisfied with the parameters they've
|
|
/// set and they want to do a final render at a higher quality and at a specific resolution
|
|
/// and save it out to a file.
|
|
/// It supports rendering either the current ember, or all of them in the opened file.
|
|
/// If a single ember is rendered, it will be saved to a single output file.
|
|
/// If multiple embers are rendered, they will all be saved to a specified directory using
|
|
/// default names.
|
|
/// The user can optionally save the Xml file with each ember.
|
|
/// They can be treated as individual images, or as an animation sequence in which case
|
|
/// motion blurring with temporal samples will be applied.
|
|
/// It keeps a pointer to the main window and the global settings object for convenience.
|
|
/// The settings used here are saved to the /finalrender portion of the settings file.
|
|
/// It has its own OpenCLWrapper member for populating the combo boxes.
|
|
/// Upon running, it will delete the main window's renderer to save memory/GPU resources and restore it to its
|
|
/// original state upon exiting.
|
|
/// This class uses a controller-based design similar to the main window.
|
|
/// </summary>
|
|
class FractoriumFinalRenderDialog : public QDialog
|
|
{
|
|
Q_OBJECT
|
|
|
|
friend Fractorium;
|
|
friend FinalRenderEmberControllerBase;
|
|
friend FinalRenderEmberController<float>;
|
|
|
|
#ifdef DO_DOUBLE
|
|
friend FinalRenderEmberController<double>;
|
|
#endif
|
|
|
|
public:
|
|
FractoriumFinalRenderDialog(FractoriumSettings* settings, QWidget* p, Qt::WindowFlags f = 0);
|
|
bool EarlyClip();
|
|
bool YAxisUp();
|
|
bool Transparency();
|
|
bool OpenCL();
|
|
bool Double();
|
|
bool SaveXml();
|
|
bool DoAll();
|
|
bool DoSequence();
|
|
bool KeepAspect();
|
|
bool ApplyToAll();
|
|
eScaleType Scale();
|
|
void Scale(eScaleType scale);
|
|
QString Ext();
|
|
QString Path();
|
|
void Path(const QString& s);
|
|
QString Prefix();
|
|
QString Suffix();
|
|
uint Current();
|
|
uint ThreadCount();
|
|
int ThreadPriority();
|
|
double WidthScale();
|
|
double HeightScale();
|
|
double Quality();
|
|
uint TemporalSamples();
|
|
uint Supersample();
|
|
uint Strips();
|
|
QList<QVariant> Devices();
|
|
FinalRenderGuiState State();
|
|
|
|
public slots:
|
|
void MoveCursorToEnd();
|
|
void OnEarlyClipCheckBoxStateChanged(int state);
|
|
void OnYAxisUpCheckBoxStateChanged(int state);
|
|
void OnTransparencyCheckBoxStateChanged(int state);
|
|
void OnOpenCLCheckBoxStateChanged(int state);
|
|
void OnDoublePrecisionCheckBoxStateChanged(int state);
|
|
void OnDoAllCheckBoxStateChanged(int state);
|
|
void OnDoSequenceCheckBoxStateChanged(int state);
|
|
void OnCurrentSpinChanged(int d);
|
|
void OnApplyAllCheckBoxStateChanged(int state);
|
|
void OnWidthScaleChanged(double d);
|
|
void OnHeightScaleChanged(double d);
|
|
void OnKeepAspectCheckBoxStateChanged(int state);
|
|
void OnScaleRadioButtonChanged(bool checked);
|
|
void OnDeviceTableCellChanged(int row, int col);
|
|
void OnDeviceTableRadioToggled(bool checked);
|
|
void OnQualityChanged(double d);
|
|
void OnTemporalSamplesChanged(int d);
|
|
void OnSupersampleChanged(int d);
|
|
void OnStripsChanged(int d);
|
|
void OnFileButtonClicked(bool checked);
|
|
void OnShowFolderButtonClicked(bool checked);
|
|
void OnExtIndexChanged(int d);
|
|
void OnPrefixChanged(const QString& s);
|
|
void OnSuffixChanged(const QString& s);
|
|
void OnRenderClicked(bool checked);
|
|
void OnCancelRenderClicked(bool checked);
|
|
virtual void reject() override;
|
|
|
|
protected:
|
|
virtual void showEvent(QShowEvent* e) override;
|
|
|
|
private:
|
|
bool CreateControllerFromGUI(bool createRenderer);
|
|
bool SetMemory();
|
|
|
|
int m_MemoryCellIndex;
|
|
int m_ItersCellIndex;
|
|
int m_PathCellIndex;
|
|
Timing m_RenderTimer;
|
|
DoubleSpinBox* m_WidthScaleSpin;
|
|
DoubleSpinBox* m_HeightScaleSpin;
|
|
DoubleSpinBox* m_QualitySpin;
|
|
SpinBox* m_TemporalSamplesSpin;
|
|
SpinBox* m_SupersampleSpin;
|
|
SpinBox* m_StripsSpin;
|
|
TwoButtonComboWidget* m_Tbcw;
|
|
QLineEdit* m_PrefixEdit;
|
|
QLineEdit* m_SuffixEdit;
|
|
FractoriumSettings* m_Settings;
|
|
Fractorium* m_Fractorium;
|
|
shared_ptr<OpenCLInfo> m_Info;
|
|
vector<OpenCLWrapper> m_Wrappers;
|
|
unique_ptr<FinalRenderEmberControllerBase> m_Controller;
|
|
Ui::FinalRenderDialog ui;
|
|
};
|