mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-06-30 13:26:02 -04:00
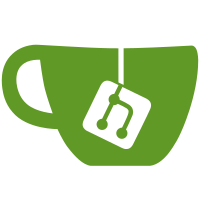
-Add variations changes to the list of functionality that can be applied to all xforms using the Select tab. -Allow for graphical affine adjustments to apply to multiple selected xforms. -Slight optimization of the pie variation. -Undo state is only saved when the render completes and the mouse buttons are released. This helps avoid intermediate steps for quickly completing renders while dragging. -Add some keyboard shortcuts for toolbar and menu items. -Make info tab tree always expanded. --Bug fixes -Make precalcs for all hypertile variations safer by using Zeps() for denominators. -Changing the current xform with more than one selected would set all xform's color index value that of the current one. -Use hard found palette path information for randoms as well. -OpenCL build and assignment errors for Z value in epispiral variation. -Unitialized local variables in hexaplay3D, crob, pRose3D. --Code changes -Change static member variables from m_ to s_. -Get rid of excessive endl and replace with "\n". -Remove old IMAGEGL2D define from before Nvidia supported OpenCL 1.2. -Remove old CriticalSection code and use std::recursive_mutex. -Make Affine2D Rotate() and RotateTrans() take radians instead of angles. -More C++11 work. -General cleanup.
148 lines
4.3 KiB
C++
148 lines
4.3 KiB
C++
#pragma once
|
|
|
|
#include "EmberDefines.h"
|
|
|
|
/// <summary>
|
|
/// Timing and CriticalSection classes.
|
|
/// </summary>
|
|
|
|
namespace EmberNs
|
|
{
|
|
/// <summary>
|
|
/// Since the algorithm is so computationally intensive, timing and benchmarking are an integral portion
|
|
/// of both the development process and the execution results. This class provides an easy way to time
|
|
/// things by simply calling its Tic() and Toc() member functions. It also assists with formatting the
|
|
/// elapsed time as a string.
|
|
/// </summary>
|
|
class EMBER_API Timing
|
|
{
|
|
public:
|
|
/// <summary>
|
|
/// Constructor that takes an optional precision argument which specifies how many digits after the decimal place should be printed for seconds.
|
|
/// As a convenience, the Tic() function is called automatically.
|
|
/// </summary>
|
|
/// <param name="precision">The precision of the seconds field of the elapsed time. Default: 2.</param>
|
|
Timing(int precision = 2)
|
|
{
|
|
m_Precision = precision;
|
|
Init();
|
|
Tic();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Set the begin time.
|
|
/// </summary>
|
|
/// <returns>The begin time cast to a double</returns>
|
|
double Tic()
|
|
{
|
|
m_BeginTime = Clock::now();
|
|
return BeginTime();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Set the end time and optionally output a string showing the elapsed time.
|
|
/// </summary>
|
|
/// <param name="str">The string to output. Default: nullptr.</param>
|
|
/// <param name="fullString">If true, output the string verbatim, else output the text " processing time: " in between str and the formatted time.</param>
|
|
/// <returns>The elapsed time in milliseconds as a double</returns>
|
|
double Toc(const char* str = nullptr, bool fullString = false)
|
|
{
|
|
m_EndTime = Clock::now();
|
|
double ms = ElapsedTime();
|
|
|
|
if (str)
|
|
{
|
|
cout << string(str) << (fullString ? "" : " processing time: ") << Format(ms) << "\n";
|
|
}
|
|
|
|
return ms;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Return the begin time as a double.
|
|
/// </summary>
|
|
/// <returns></returns>
|
|
double BeginTime() const { return static_cast<double>(m_BeginTime.time_since_epoch().count()); }
|
|
|
|
/// <summary>
|
|
/// Return the end time as a double.
|
|
/// </summary>
|
|
/// <returns></returns>
|
|
double EndTime() const { return static_cast<double>(m_EndTime.time_since_epoch().count()); }
|
|
|
|
/// <summary>
|
|
/// Return the elapsed time in milliseconds.
|
|
/// </summary>
|
|
/// <returns>The elapsed time in milliseconds as a double</returns>
|
|
double ElapsedTime() const
|
|
{
|
|
duration<double> elapsed = duration_cast<milliseconds, Clock::rep, Clock::period>(m_EndTime - m_BeginTime);
|
|
return elapsed.count() * 1000.0;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Formats a specified milliseconds value as a string.
|
|
/// This uses some intelligence to determine what to return depending on how much time has elapsed.
|
|
/// Days, hours and minutes are only included if 1 or more of them has elapsed. Seconds are always
|
|
/// included as a decimal value with the precision the user specified in the constructor.
|
|
/// </summary>
|
|
/// <param name="ms">The ms</param>
|
|
/// <returns>The formatted string</returns>
|
|
string Format(double ms) const
|
|
{
|
|
stringstream ss;
|
|
double x = ms / 1000;
|
|
double secs = fmod(x, 60);
|
|
x /= 60;
|
|
double mins = fmod(x, 60);
|
|
x /= 60;
|
|
double hours = fmod(x, 24);
|
|
x /= 24;
|
|
double days = x;
|
|
|
|
if (days >= 1)
|
|
ss << static_cast<int>(days) << "d ";
|
|
|
|
if (hours >= 1)
|
|
ss << static_cast<int>(hours) << "h ";
|
|
|
|
if (mins >= 1)
|
|
ss << static_cast<int>(mins) << "m ";
|
|
|
|
ss << std::fixed << std::setprecision(m_Precision) << secs << "s";
|
|
return ss.str();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Return the number of cores in the system.
|
|
/// </summary>
|
|
/// <returns>The number of cores in the system</returns>
|
|
static uint ProcessorCount()
|
|
{
|
|
Init();
|
|
return m_ProcessorCount;
|
|
}
|
|
|
|
private:
|
|
/// <summary>
|
|
/// Query and store the performance info of the system.
|
|
/// Since it will never change it only needs to be queried once.
|
|
/// This is achieved by keeping static state and performance variables.
|
|
/// </summary>
|
|
static void Init()
|
|
{
|
|
if (!m_TimingInit)
|
|
{
|
|
m_ProcessorCount = thread::hardware_concurrency();
|
|
m_TimingInit = true;
|
|
}
|
|
}
|
|
|
|
int m_Precision;//How many digits after the decimal place to print for seconds.
|
|
time_point<Clock> m_BeginTime;//The start of the timing, set with Tic().
|
|
time_point<Clock> m_EndTime;//The end of the timing, set with Toc().
|
|
static bool m_TimingInit;//Whether the performance info has bee queried.
|
|
static uint m_ProcessorCount;//The number of cores on the system, set in Init().
|
|
};
|
|
}
|