mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-02 22:34:52 -04:00
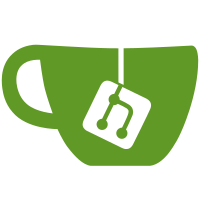
-Add two new variations, hyperbolic and hypershift2. -Allow for animating final xforms. -More detailed diagnostics when any action in the OpenCL renderer fails. -Allow for creating an OpenCL renderer which does not share a texture with the main window, and instead manually copies its final output image from GPU to CPU then back to GPU. --Bug fixes -Text was not properly being copied out of the Info | Bounds text box. --Code changes -Remove Renderer::AccumulatorToFinalImage(v4F* pixels, size_t finalOffset), it's no longer needed or makes sense. -Controllers no longer keep track of shared status, it's kept inside the renderers. -Make getter functions in FractoriumOptionsDialog be public.
48 lines
1.0 KiB
C++
48 lines
1.0 KiB
C++
#pragma once
|
|
|
|
#include "EmberCLPch.h"
|
|
#include "OpenCLWrapper.h"
|
|
#include "IterOpenCLKernelCreator.h"
|
|
|
|
/// <summary>
|
|
/// RendererClDevice class.
|
|
/// </summary>
|
|
|
|
namespace EmberCLns
|
|
{
|
|
/// <summary>
|
|
/// Class to manage a device that does the iteration portion of
|
|
/// the rendering process. Having a separate class for this purpose
|
|
/// enables multi-GPU support.
|
|
/// </summary>
|
|
class EMBERCL_API RendererClDevice : public EmberReport
|
|
{
|
|
public:
|
|
RendererClDevice(size_t platform, size_t device, bool shared);
|
|
bool Init();
|
|
bool Ok() const;
|
|
bool Shared() const;
|
|
bool Nvidia() const;
|
|
size_t WarpSize() const;
|
|
size_t PlatformIndex() const;
|
|
size_t DeviceIndex() const;
|
|
|
|
//Public virtual functions overridden from base classes.
|
|
virtual void ClearErrorReport() override;
|
|
virtual string ErrorReportString() override;
|
|
virtual vector<string> ErrorReport() override;
|
|
|
|
size_t m_Calls;
|
|
OpenCLWrapper m_Wrapper;
|
|
|
|
private:
|
|
bool m_Init;
|
|
bool m_Shared;
|
|
bool m_NVidia;
|
|
size_t m_WarpSize;
|
|
size_t m_PlatformIndex;
|
|
size_t m_DeviceIndex;
|
|
shared_ptr<OpenCLInfo> m_Info;
|
|
};
|
|
}
|