mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-21 21:20:07 -05:00
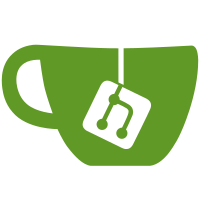
Allow for setting rendering thread priorities from the command line and final render dialog. Currently only implemented on Windows. Show estimated time left on the final render dialog. Sort palette list by name, instead of by index in the palette file. Sorting can be flipped by clicking the column headers of the palette table. --Code changes Remove unnecessary connect() statement in Variations tab.
38 lines
811 B
C++
38 lines
811 B
C++
#pragma once
|
|
|
|
#include "FractoriumPch.h"
|
|
|
|
/// <summary>
|
|
/// PaletteTableWidgetItem class.
|
|
/// </summary>
|
|
|
|
/// <summary>
|
|
/// A thin derivation of QTableWidgetItem which keeps a pointer to a palette object.
|
|
/// The lifetime of the palette object must be greater than or equal to
|
|
/// the lifetime of this object.
|
|
/// </summary>
|
|
class PaletteTableWidgetItemBase : public QTableWidgetItem
|
|
{
|
|
public:
|
|
PaletteTableWidgetItemBase()
|
|
{
|
|
}
|
|
|
|
virtual size_t Index() const { return 0; }
|
|
};
|
|
|
|
template <typename T>
|
|
class PaletteTableWidgetItem : public PaletteTableWidgetItemBase
|
|
{
|
|
public:
|
|
PaletteTableWidgetItem(Palette<T>* palette)
|
|
: m_Palette(palette)
|
|
{
|
|
}
|
|
|
|
virtual size_t Index() const override { return m_Palette->m_Index; }
|
|
Palette<T>* GetPalette() const { return m_Palette; }
|
|
|
|
private:
|
|
Palette<T>* m_Palette;
|
|
}; |