mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-11 18:54:53 -04:00
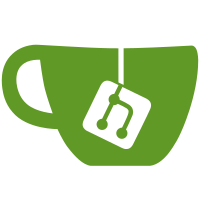
-Remove the option --intpalette to format the palette in the xml as ints. If they are not hex formatted, then they should always be float. This option was pointless. -Cleanup some options text for the command line programs. -Allow for dragging around flames in the library tab. This is useful for setting up the order of an animation. -Make the opening of large files in Fractorium much more efficient when not-appending. -Make the opening of large files in all EmberRender and EmberAnimate more efficient. -Better error reporting when opening files. --Bug fixes -Get rid of leftover artifacts that would appear on preview thumbnails when either switching SP/DP or re-rendering previews. -Filename extension was not being appended on Linux when saving as Xml, thus making it impossible to drag that file back in becase drop is filtered on extension. --Code changes -Move GCC compiler spec to C++14. Building with 5.3 now on linux. -Use inline member data initializers. -Make a #define for static for use in Utils.h to make things a little cleaner. -Make various functions able to take arbitrary collections as their parameters rather than just vectors. -Make library collection a list rather than vector. This alleviates the need to re-sync pointers whenever the collection changes. -Subclass QTreeWidget for the library tree. Two new files added for this. -Remove all usage of #ifdef ROW_ONLY_DE in DEOpenCLKernelCreator, it was never used. -Add move constructor and assignment operator to EmberFile. -Add the ability to use a pointer to outside memory in the renderer for the vector of Ember<T>. -Make a lot more functions const where they should be.
146 lines
3.8 KiB
C++
146 lines
3.8 KiB
C++
#pragma once
|
|
|
|
#include "EmberDefines.h"
|
|
|
|
namespace EmberNs
|
|
{
|
|
/// <summary>
|
|
/// Thin derivation of pair<eEmberMotionParam, T> for the element type
|
|
/// of EmberMotion<T>::m_MotionParams to allow for copying vectors
|
|
/// of different types of T.
|
|
/// Template argument expected to be float or double.
|
|
/// </summary>
|
|
template <typename T>
|
|
class EMBER_API MotionParam : public pair <eEmberMotionParam, T>
|
|
{
|
|
public:
|
|
/// <summary>
|
|
/// Default constructor, which calls the base, which sets first and second to their defaults.
|
|
/// </summary>
|
|
MotionParam() = default;
|
|
|
|
/// <summary>
|
|
/// Member-wise constructor.
|
|
/// </summary>
|
|
/// <param name="e">The eEmberMotionParam value to assign to first</param>
|
|
/// <param name="t">The T value to assign to second</param>
|
|
MotionParam(eEmberMotionParam e, T t)
|
|
: pair<eEmberMotionParam, T>(e, t)
|
|
{
|
|
}
|
|
|
|
/// <summary>
|
|
/// Default copy constructor.
|
|
/// </summary>
|
|
/// <param name="other">The MotionParam object to copy</param>
|
|
MotionParam(const MotionParam<T>& other)
|
|
: pair <eEmberMotionParam, T>()
|
|
{
|
|
operator=<T>(other);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Copy constructor to copy a MotionParam object of type U.
|
|
/// </summary>
|
|
/// <param name="other">The MotionParam object to copy</param>
|
|
template <typename U>
|
|
MotionParam(const MotionParam<U>& other)
|
|
{
|
|
operator=<U>(other);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Default assignment operator.
|
|
/// </summary>
|
|
/// <param name="other">The MotionParam object to copy</param>
|
|
MotionParam<T>& operator = (const MotionParam<T>& other)
|
|
{
|
|
if (this != &other)
|
|
MotionParam<T>::operator=<T>(other);
|
|
|
|
return *this;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Assignment operator to assign a MotionParam object of type U.
|
|
/// </summary>
|
|
/// <param name="other">The MotionParam object to copy.</param>
|
|
/// <returns>Reference to updated self</returns>
|
|
template <typename U>
|
|
MotionParam& operator = (const MotionParam<U>& other)
|
|
{
|
|
this->first = other.first;
|
|
this->second = T(other.second);
|
|
return *this;
|
|
}
|
|
};
|
|
|
|
/// <summary>
|
|
/// EmberMotion elements allow for motion of the flame parameters such as zoom, yaw, pitch and friends
|
|
/// The values in these elements can be used to modify flame parameters during rotation in much the same
|
|
/// way as motion elements on xforms do.
|
|
/// Template argument expected to be float or double.
|
|
/// </summary>
|
|
template <typename T>
|
|
class EMBER_API EmberMotion
|
|
{
|
|
public:
|
|
/// <summary>
|
|
/// Default constructor to initialize motion freq and offset to 0 and the motion func to SIN.
|
|
/// </summary>
|
|
EmberMotion() = default;
|
|
~EmberMotion() = default;
|
|
|
|
/// <summary>
|
|
/// Default copy constructor.
|
|
/// </summary>
|
|
/// <param name="other">The EmberMotion object to copy</param>
|
|
EmberMotion(const EmberMotion<T>& other)
|
|
{
|
|
operator=<T>(other);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Copy constructor to copy a EmberMotion object of type U.
|
|
/// </summary>
|
|
/// <param name="other">The EmberMotion object to copy</param>
|
|
template <typename U>
|
|
EmberMotion(const EmberMotion<U>& other)
|
|
{
|
|
operator=<U>(other);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Default assignment operator.
|
|
/// </summary>
|
|
/// <param name="other">The EmberMotion object to copy</param>
|
|
EmberMotion<T>& operator = (const EmberMotion<T>& other)
|
|
{
|
|
if (this != &other)
|
|
EmberMotion<T>::operator=<T>(other);
|
|
|
|
return *this;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Assignment operator to assign a EmberMotion object of type U.
|
|
/// </summary>
|
|
/// <param name="other">The EmberMotion object to copy.</param>
|
|
/// <returns>Reference to updated self</returns>
|
|
template <typename U>
|
|
EmberMotion& operator = (const EmberMotion<U>& other)
|
|
{
|
|
CopyCont(m_MotionParams, other.m_MotionParams);
|
|
m_MotionFunc = other.m_MotionFunc;
|
|
m_MotionFreq = T(other.m_MotionFreq);
|
|
m_MotionOffset = T(other.m_MotionOffset);
|
|
return *this;
|
|
}
|
|
|
|
T m_MotionFreq = 0;
|
|
T m_MotionOffset = 0;
|
|
eMotion m_MotionFunc = eMotion::MOTION_SIN;
|
|
vector<MotionParam<T>> m_MotionParams;
|
|
};
|
|
}
|