mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-03 14:54:52 -04:00
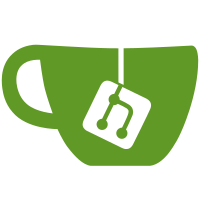
-Add a palette editor. -Add support for reading .ugr/.gradient/.gradients palette files. -Allow toggling on spinners whose minimum value is not zero. -Allow toggling display of image, affines and grid. -Add new variations: cylinder2, circlesplit, tile_log, truchet_fill, waves2_radial. --Bug fixes -cpow2 was wrong. -Palettes with rapid changes in color would produce slightly different outputs from Apo/Chaotica. This was due to a long standing bug from flam3. -Use exec() on Apple and show() on all other OSes for dialog boxes. -Trying to render a sequence with no frames would crash. -Selecting multiple xforms and rotating them would produce the wrong rotation. -Better handling when parsing flames using different encoding, such as unicode and UTF-8. -Switching between SP/DP didn't reselect the selected flame in the Library tab. --Code changes -Make all types concerning palettes be floats, including PaletteTableWidgetItem. -PaletteTableWidgetItem is no longer templated because all palettes are float. -Include the source colors for user created gradients. -Change parallel_for() calls to work with very old versions of TBB which are lingering on some systems. -Split conditional out of accumulation loop on the CPU for better performance. -Vectorize summing when doing density filter for better performance. -Make all usage of palettes be of type float, double is pointless. -Allow palettes to reside in multiple folders, while ensuring only one of each name is added. -Refactor some palette path searching code. -Make ReadFile() throw and catch an exception if the file operation fails. -A little extra safety in foci and foci3D with a call to Zeps(). -Cast to (real_t) in the OpenCL string for the w variation, which was having trouble compiling on Mac. -Fixing missing comma between paths in InitPaletteList(). -Move Xml and PaletteList classes into cpp to shorten build times when working on them. -Remove default param values for IterOpenCLKernelCreator<T>::SharedDataIndexDefines in cpp file. -Change more NULL to nullptr.
197 lines
5.8 KiB
C++
197 lines
5.8 KiB
C++
#include "FractoriumPch.h"
|
|
#include "Fractorium.h"
|
|
#include "QssDialog.h"
|
|
|
|
/// <summary>
|
|
/// Initialize the toolbar UI.
|
|
/// </summary>
|
|
void Fractorium::InitToolbarUI()
|
|
{
|
|
auto clGroup = new QActionGroup(this);
|
|
clGroup->addAction(ui.ActionCpu);
|
|
clGroup->addAction(ui.ActionCL);
|
|
auto spGroup = new QActionGroup(this);
|
|
spGroup->addAction(ui.ActionSP);
|
|
spGroup->addAction(ui.ActionDP);
|
|
SyncOptionsToToolbar();
|
|
ui.ActionDrawImage->setChecked(true);
|
|
connect(ui.ActionCpu, SIGNAL(triggered(bool)), this, SLOT(OnActionCpu(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionCL, SIGNAL(triggered(bool)), this, SLOT(OnActionCL(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionSP, SIGNAL(triggered(bool)), this, SLOT(OnActionSP(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionDP, SIGNAL(triggered(bool)), this, SLOT(OnActionDP(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionStyle, SIGNAL(triggered(bool)), this, SLOT(OnActionStyle(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionStartStopRenderer, SIGNAL(triggered(bool)), this, SLOT(OnActionStartStopRenderer(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionDrawXforms, SIGNAL(triggered(bool)), this, SLOT(OnActionDrawXforms(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionDrawImage, SIGNAL(triggered(bool)), this, SLOT(OnActionDrawImage(bool)), Qt::QueuedConnection);
|
|
connect(ui.ActionDrawGrid, SIGNAL(triggered(bool)), this, SLOT(OnActionDrawGrid(bool)), Qt::QueuedConnection);
|
|
}
|
|
|
|
/// <summary>
|
|
/// GUI wrapper functions, getters only.
|
|
/// </summary>
|
|
|
|
bool Fractorium::DrawXforms() { return ui.ActionDrawXforms->isChecked(); }
|
|
bool Fractorium::DrawImage() { return ui.ActionDrawImage->isChecked(); }
|
|
bool Fractorium::DrawGrid() { return ui.ActionDrawGrid->isChecked(); }
|
|
|
|
/// <summary>
|
|
/// Called when the CPU render option on the toolbar is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, action only taken if true.</param>
|
|
void Fractorium::OnActionCpu(bool checked)
|
|
{
|
|
if (checked && m_Settings->OpenCL())
|
|
{
|
|
m_Settings->OpenCL(false);
|
|
ShutdownAndRecreateFromOptions();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the OpenCL render option on the toolbar is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, action only taken if true.</param>
|
|
void Fractorium::OnActionCL(bool checked)
|
|
{
|
|
if (checked && !m_Settings->OpenCL())
|
|
{
|
|
m_Settings->OpenCL(true);
|
|
ShutdownAndRecreateFromOptions();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the single precision render option on the toolbar is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, action only taken if true.</param>
|
|
void Fractorium::OnActionSP(bool checked)
|
|
{
|
|
if (checked && m_Settings->Double())
|
|
{
|
|
m_Settings->Double(false);
|
|
ShutdownAndRecreateFromOptions();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the double precision render option on the toolbar is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, action only taken if true.</param>
|
|
void Fractorium::OnActionDP(bool checked)
|
|
{
|
|
if (checked && !m_Settings->Double())
|
|
{
|
|
m_Settings->Double(true);
|
|
ShutdownAndRecreateFromOptions();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the show style button is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Ignored</param>
|
|
void Fractorium::OnActionStyle(bool checked)
|
|
{
|
|
#ifdef __APPLE__
|
|
m_QssDialog->exec();
|
|
#else
|
|
m_QssDialog->show();
|
|
#endif
|
|
}
|
|
|
|
/// <summary>
|
|
/// Called when the start/stop renderer button is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, stop renderer if true, else start.</param>
|
|
void Fractorium::OnActionStartStopRenderer(bool checked)
|
|
{
|
|
EnableRenderControls(!checked);
|
|
|
|
if (checked)
|
|
{
|
|
m_Controller->StopRenderTimer(true);
|
|
ui.ActionStartStopRenderer->setToolTip("Start Renderer");
|
|
ui.ActionStartStopRenderer->setIcon(QIcon(":/Fractorium/Icons/control.png"));
|
|
}
|
|
else
|
|
{
|
|
m_Controller->StartRenderTimer();
|
|
ui.ActionStartStopRenderer->setToolTip("Stop Renderer");
|
|
ui.ActionStartStopRenderer->setIcon(QIcon(":/Fractorium/Icons/control-stop-square.png"));
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Toggle whether to show the affines.
|
|
/// Called when the editor image button is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, show editor if true, else hide.</param>
|
|
void Fractorium::OnActionDrawXforms(bool checked)
|
|
{
|
|
if (!ui.ActionDrawImage->isChecked() && !ui.ActionDrawXforms->isChecked())
|
|
ui.ActionDrawImage->setChecked(true);
|
|
|
|
ui.GLDisplay->update();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Toggle whether to show the image.
|
|
/// Called when the image button is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, show image if true, else hide.</param>
|
|
void Fractorium::OnActionDrawImage(bool checked)
|
|
{
|
|
if (!ui.ActionDrawImage->isChecked() && !ui.ActionDrawXforms->isChecked())
|
|
ui.ActionDrawXforms->setChecked(true);
|
|
|
|
ui.GLDisplay->update();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Toggle whether to show the grid.
|
|
/// Called when the grid image button is clicked.
|
|
/// </summary>
|
|
/// <param name="checked">Check state, show grid if true, else hide.</param>
|
|
void Fractorium::OnActionDrawGrid(bool checked)
|
|
{
|
|
ui.GLDisplay->update();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Sync options data to the check state of the toolbar buttons.
|
|
/// This does not trigger a clicked() event.
|
|
/// </summary>
|
|
void Fractorium::SyncOptionsToToolbar()
|
|
{
|
|
static bool openCL = !m_Info->Devices().empty();
|
|
|
|
if (!openCL)
|
|
{
|
|
ui.ActionCL->setEnabled(false);
|
|
}
|
|
|
|
if (openCL && m_Settings->OpenCL())
|
|
{
|
|
ui.ActionCpu->setChecked(false);
|
|
ui.ActionCL->setChecked(true);
|
|
}
|
|
else
|
|
{
|
|
ui.ActionCpu->setChecked(true);
|
|
ui.ActionCL->setChecked(false);
|
|
}
|
|
|
|
if (m_Settings->Double())
|
|
{
|
|
ui.ActionSP->setChecked(false);
|
|
ui.ActionDP->setChecked(true);
|
|
}
|
|
else
|
|
{
|
|
ui.ActionSP->setChecked(true);
|
|
ui.ActionDP->setChecked(false);
|
|
}
|
|
|
|
ui.ActionDrawGrid->setChecked(m_Settings->ShowGrid());
|
|
ui.ActionDrawXforms->setChecked(m_Settings->ShowXforms());
|
|
} |