mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-11 18:54:53 -04:00
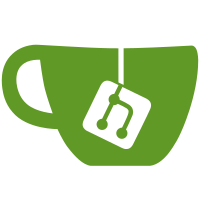
-Give tabs a height of 4px in the qss files. Looks a little large on 4k screens, but just right on HD screens which are much more common. -Allow for styling of zero and non-zero variation tree nodes via qss. -Allow for toggling whether to interpolate between colors in the palette editor, or to do hard cuts between colors. -Allow for adjusting spinner values with the + = or up arrow keys to increase, and - _ or down arrow keys to decrease. -Allow for responding to global presses of + = and - _ to cycle up or down to specify which xform is set as the current one. -Allow for adding "layers" via xaos which will add a user-specified number of xforms, and set certain xaos values to 0 or 1. -Add a new menu item under the Edit menu to copy the OpenCL iteration kernel source to the clipboard. -Show text on the status bar which indicates that an OpenCL kernel compilation is taking place. -Show xform name on xform combo box when expanded. Adjust size to fit all names. -Draw post affine circles using dashed lines. -Prevent QSS dialog from styling its editor, which makes it easier to see text when creating styles which have custom colors for text boxes. --Bug fixes -Fix up some table layouts which seemed to have regressed/decayed over time for reasons unknown. -Using undo/redo would create a new flame in the library every time. -Solo was not being preserved when using undo/redo. --Code changes -Make the solo flag be a part of the flame data now. -Fix some tabification in the OpenCL code for EllipticVariation. -Fix tabification in the varState code for OpenCL. -Add an event called m_CompileBegun to RendererCL that is called right before an OpenCL compile is begun. --This required making RendererCLBase not a pure virtual base class. Member functions just return defaults. -Filter key presses on main window to only process the third one. This is due to Qt triggering three events for every key press. -The palette preview table was installing an event filter for seemingly no reason. Remove it. -Mark certain virtual functions as override in SpinBox and DoubleSpinBox.
63 lines
1.6 KiB
C++
63 lines
1.6 KiB
C++
#pragma once
|
|
|
|
#include "FractoriumPch.h"
|
|
#include "DoubleSpinBox.h"
|
|
|
|
/// <summary>
|
|
/// SpinBox class.
|
|
/// </summary>
|
|
|
|
/// <summary>
|
|
/// A derivation to prevent the spin box from selecting its own text
|
|
/// when editing. Also to prevent multiple spin boxes from all having
|
|
/// selected text at once.
|
|
/// </summary>
|
|
class SpinBox : public QSpinBox
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
explicit SpinBox(QWidget* p = nullptr, int height = 16, int step = 1);
|
|
virtual ~SpinBox() { }
|
|
void SetValueStealth(int d);
|
|
void SetValueStealth(size_t d);
|
|
void DoubleClick(bool b);
|
|
void DoubleClickLowVal(int val);
|
|
int DoubleClickLowVal();
|
|
void DoubleClickZero(int val);
|
|
int DoubleClickZero();
|
|
void DoubleClickNonZero(int val);
|
|
int DoubleClickNonZero();
|
|
void SmallStep(int step);
|
|
QLineEdit* lineEdit();
|
|
std::function<void(SpinBox*, int)> m_DoubleClickZeroEvent = [&](SpinBox*, int) {};
|
|
std::function<void(SpinBox*, int)> m_DoubleClickNonZeroEvent = [&](SpinBox*, int) {};
|
|
|
|
public slots:
|
|
void onSpinBoxValueChanged(int i);
|
|
void OnTimeout();
|
|
|
|
protected:
|
|
virtual bool eventFilter(QObject* o, QEvent* e) override;
|
|
virtual void keyPressEvent(QKeyEvent* event) override;
|
|
virtual void focusInEvent(QFocusEvent* e) override;
|
|
virtual void focusOutEvent(QFocusEvent* e) override;
|
|
virtual void enterEvent(QEvent* e) override;
|
|
virtual void leaveEvent(QEvent* e) override;
|
|
|
|
private:
|
|
void StartTimer();
|
|
void StopTimer();
|
|
|
|
bool m_DoubleClick;
|
|
int m_DoubleClickLowVal;
|
|
int m_DoubleClickNonZero;
|
|
int m_DoubleClickZero;
|
|
int m_Step;
|
|
int m_SmallStep;
|
|
QPoint m_MouseDownPoint;
|
|
QPoint m_MouseMovePoint;
|
|
shared_ptr<FractoriumSettings> m_Settings;
|
|
static QTimer s_Timer;
|
|
};
|