mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-22 13:40:06 -05:00
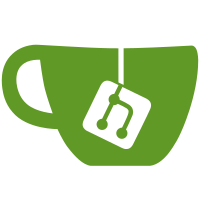
-Add a palette editor. -Add support for reading .ugr/.gradient/.gradients palette files. -Allow toggling on spinners whose minimum value is not zero. -Allow toggling display of image, affines and grid. -Add new variations: cylinder2, circlesplit, tile_log, truchet_fill, waves2_radial. --Bug fixes -cpow2 was wrong. -Palettes with rapid changes in color would produce slightly different outputs from Apo/Chaotica. This was due to a long standing bug from flam3. -Use exec() on Apple and show() on all other OSes for dialog boxes. -Trying to render a sequence with no frames would crash. -Selecting multiple xforms and rotating them would produce the wrong rotation. -Better handling when parsing flames using different encoding, such as unicode and UTF-8. -Switching between SP/DP didn't reselect the selected flame in the Library tab. --Code changes -Make all types concerning palettes be floats, including PaletteTableWidgetItem. -PaletteTableWidgetItem is no longer templated because all palettes are float. -Include the source colors for user created gradients. -Change parallel_for() calls to work with very old versions of TBB which are lingering on some systems. -Split conditional out of accumulation loop on the CPU for better performance. -Vectorize summing when doing density filter for better performance. -Make all usage of palettes be of type float, double is pointless. -Allow palettes to reside in multiple folders, while ensuring only one of each name is added. -Refactor some palette path searching code. -Make ReadFile() throw and catch an exception if the file operation fails. -A little extra safety in foci and foci3D with a call to Zeps(). -Cast to (real_t) in the OpenCL string for the w variation, which was having trouble compiling on Mac. -Fixing missing comma between paths in InitPaletteList(). -Move Xml and PaletteList classes into cpp to shorten build times when working on them. -Remove default param values for IterOpenCLKernelCreator<T>::SharedDataIndexDefines in cpp file. -Change more NULL to nullptr.
83 lines
2.9 KiB
C++
83 lines
2.9 KiB
C++
#pragma once
|
|
|
|
#include "FractoriumEmberController.h"
|
|
|
|
/// <summary>
|
|
/// GLWidget class.
|
|
/// </summary>
|
|
|
|
class Fractorium;//Forward declaration since Fractorium uses this widget.
|
|
class GLEmberControllerBase;
|
|
template<typename T> class GLEmberController;
|
|
template<typename T> class FractoriumEmberController;
|
|
|
|
/// <summary>
|
|
/// The main drawing area.
|
|
/// This uses the Qt wrapper around OpenGL to draw the output of the render to a texture whose
|
|
/// size matches the size of the window.
|
|
/// On top of that, the circles that represent the pre and post affine transforms for each xform are drawn.
|
|
/// Based on values specified on the GUI, it will either draw the presently selected xform, or all of them.
|
|
/// It can show/hide pre/post affine as well.
|
|
/// The currently selected xform is drawn with a circle around it, with all others only showing their axes.
|
|
/// The current xform is set by either clicking on it, or by changing the index of the xforms combo box on the main window.
|
|
/// A problem here is that all drawing is done using the legacy OpenGL fixed function pipeline which is deprecated
|
|
/// and even completely disabled on Mac OS. This will need to be replaced with shader programs for every draw operation.
|
|
/// Since this window has to know about various states of the renderer and the main window, it retains pointers to
|
|
/// the main window and several of its members.
|
|
/// This class uses a controller-based design similar to the main window.
|
|
/// </summary>
|
|
class GLWidget : public QOpenGLWidget, protected QOpenGLFunctions_2_0//QOpenGLFunctions_3_2_Compatibility//QOpenGLFunctions_3_2_Core//, protected QOpenGLFunctions
|
|
{
|
|
Q_OBJECT
|
|
|
|
friend Fractorium;
|
|
friend FractoriumEmberController<float>;
|
|
friend GLEmberControllerBase;
|
|
friend GLEmberController<float>;
|
|
|
|
#ifdef DO_DOUBLE
|
|
friend GLEmberController<double>;
|
|
friend FractoriumEmberController<double>;
|
|
#endif
|
|
|
|
public:
|
|
GLWidget(QWidget* p = nullptr);
|
|
~GLWidget();
|
|
void InitGL();
|
|
void DrawQuad();
|
|
void SetMainWindow(Fractorium* f);
|
|
bool Init();
|
|
bool Drawing();
|
|
GLint MaxTexSize();
|
|
GLuint OutputTexID();
|
|
|
|
protected:
|
|
virtual void initializeGL() override;
|
|
virtual void paintGL() override;
|
|
virtual void keyPressEvent(QKeyEvent* e) override;
|
|
virtual void keyReleaseEvent(QKeyEvent* e) override;
|
|
virtual void mousePressEvent(QMouseEvent* e) override;
|
|
virtual void mouseReleaseEvent(QMouseEvent* e) override;
|
|
virtual void mouseMoveEvent(QMouseEvent* e) override;
|
|
virtual void wheelEvent(QWheelEvent* e) override;
|
|
|
|
private:
|
|
void SetDimensions(int w, int h);
|
|
bool Allocate(bool force = false);
|
|
bool Deallocate();
|
|
void SetViewport();
|
|
void DrawUnitSquare();
|
|
void DrawAffineHelper(int index, bool selected, bool pre, bool final, bool background);
|
|
GLEmberControllerBase* GLController();
|
|
|
|
bool m_Init = false;
|
|
bool m_Drawing = false;
|
|
GLint m_MaxTexSize = 16384;
|
|
GLint m_TexWidth = 0;
|
|
GLint m_TexHeight = 0;
|
|
GLint m_ViewWidth = 0;
|
|
GLint m_ViewHeight = 0;
|
|
GLuint m_OutputTexID = 0;
|
|
Fractorium* m_Fractorium = nullptr;
|
|
};
|