mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-22 05:30:06 -05:00
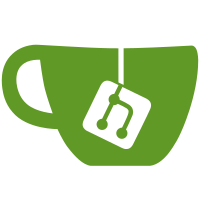
--User changes -Add many tooltips to help clarify functionality. -Select multiple flames in library for del/move. Still only one allowed to be set as the current. -Show checkbox for current flame. Remember this is not necessarily what's selected. -User can now drag a square to select xforms, which keeps in sync with checkboxes. -Remove --nframes from command line. Replace with new params: --loopframes, --interpframes, --interploops. -Add two new options to EmberGenome: --cwloops --cwinterploops to specify whether rotation should go clockwise instead of the default counter clockwise. -Add these to Fractorium as checkboxes. -Apply All now also works for toggling animate flag on xforms. -Options dialog now allows user to set whether double click toggles spinners, or right click does. --Bug fixes -Selecting final and non-final xforms, and then dragging the non-final did not drag the final with it. -Selecting all xforms when a final was present, then deleting crashed the program. -Remove support for ppm files in the command line programs, it's an outdated format. -Switching between SP and DP kept reapplying the palette adjustments. --Code changes -Move build system to Visual Studio 2015 and Qt 5.6. -SSE used during addition of points to the histogram. -Remove last remnants of old flam3 C code and replace with C++. -Remove unused code involving tbb::task_group. -Make settings object a global shared_ptr singleton, so it doesn't have to be passed around.
52 lines
1.6 KiB
C++
52 lines
1.6 KiB
C++
#pragma once
|
|
|
|
#include "ui_VariationsDialog.h"
|
|
#include "FractoriumSettings.h"
|
|
|
|
/// <summary>
|
|
/// FractoriumVariationsDialog class.
|
|
/// </summary>
|
|
|
|
/// <summary>
|
|
/// The variations filter dialog displays several columns
|
|
/// with the different types of variations shown as checkboxes.
|
|
/// This is used to filter the variations that are shown in the main window
|
|
/// because the list is very long.
|
|
/// The results are stored in a map and returned.
|
|
/// These are used in conjunction with the filter edit box to filter what's shown.
|
|
/// </summary>
|
|
class FractoriumVariationsDialog : public QDialog
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
FractoriumVariationsDialog(QWidget* p = nullptr, Qt::WindowFlags f = nullptr);
|
|
void ForEachCell(std::function<void(QTableWidgetItem* cb)> func);
|
|
void ForEachSelectedCell(std::function<void(QTableWidgetItem* cb)> func);
|
|
void SyncSettings();
|
|
const QMap<QString, QVariant>& Map();
|
|
|
|
public slots:
|
|
void OnSelectAllButtonClicked(bool checked);
|
|
void OnInvertSelectionButtonClicked(bool checked);
|
|
void OnSelectNoneButtonClicked(bool checked);
|
|
void OnSelectionCheckBoxStateChanged(int i);
|
|
void OnVariationsTableItemChanged(QTableWidgetItem* item);
|
|
virtual void accept() override;
|
|
virtual void reject() override;
|
|
|
|
protected:
|
|
virtual void showEvent(QShowEvent* e) override;
|
|
|
|
private:
|
|
void ClearTypesStealth();
|
|
void DataToGui();
|
|
void GuiToData();
|
|
void Populate();
|
|
void SetCheckFromMap(QTableWidgetItem* cb, const Variation<float>* var);
|
|
shared_ptr<VariationList<float>> m_VariationList;
|
|
vector<QCheckBox*> m_CheckBoxes;
|
|
QMap<QString, QVariant> m_Vars;
|
|
shared_ptr<FractoriumSettings> m_Settings;
|
|
Ui::VariationsDialog ui;
|
|
};
|