mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-21 05:00:06 -05:00
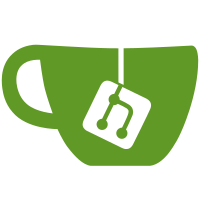
-Add new preset dimensions to the right click menu of the width and height fields in the editor. -Change QSS stylesheets to properly handle tabs. -Make tabs rectangular by default. For some reason, they had always been triangular. --Bug fixes -Incremental rendering times in the editor were wrong. --Code changes -Migrate to Qt6. There is probably more work to be done here. -Migrate to VS2022. -Migrate to Wix 4 installer. -Change installer to install to program files for all users. -Fix many VS2022 code analysis warnings. -No longer use byte typedef, because std::byte is now a type. Revert all back to unsigned char. -Upgrade OpenCL headers to version 3.0 and keep locally now rather than trying to look for system files. -No longer link to Nvidia or AMD specific OpenCL libraries. Use the generic installer located at OCL_ROOT too. -Add the ability to change OpenCL grid dimensions. This was attempted for investigating possible performance improvments, but made no difference. This has not been verified on Linux or Mac yet.
91 lines
3.0 KiB
C++
91 lines
3.0 KiB
C++
#pragma once
|
|
|
|
#include "FractoriumPch.h"
|
|
#include "DoubleSpinBox.h"
|
|
|
|
/// <summary>
|
|
/// VariationTreeWidgetItem class.
|
|
/// </summary>
|
|
|
|
/// <summary>
|
|
/// A derivation of QTreeWidgetItem which helps us with sorting.
|
|
/// This is used when the user chooses to sort the variations tree
|
|
/// by index or by weight. It supports weights less than, equal to, or
|
|
/// greater than zero.
|
|
/// </summary>
|
|
class VariationTreeWidgetItem : public QTreeWidgetItem
|
|
{
|
|
public:
|
|
/// <summary>
|
|
/// Constructor that takes a pointer to a QTreeWidget as the parent
|
|
/// and passes it to the base.
|
|
/// </summary>
|
|
/// <param name="id">The ID of the variation this widget will represent</param>
|
|
/// <param name="p">The parent widget</param>
|
|
VariationTreeWidgetItem(eVariationId id, QTreeWidget* p = nullptr)
|
|
: QTreeWidgetItem(p)
|
|
{
|
|
m_Id = id;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Constructor that takes a pointer to a QTreeWidgetItem as the parent
|
|
/// and passes it to the base.
|
|
/// This is used for making sub items for parametric variation parameters.
|
|
/// </summary>
|
|
/// <param name="id">The ID of the variation this widget will represent</param>
|
|
/// <param name="p">The parent widget</param>
|
|
VariationTreeWidgetItem(eVariationId id, QTreeWidgetItem* p = 0)
|
|
: QTreeWidgetItem(p)
|
|
{
|
|
m_Id = id;
|
|
}
|
|
|
|
//virtual ~VariationTreeWidgetItem() { }
|
|
eVariationId Id() const { return m_Id; }
|
|
|
|
private:
|
|
/// <summary>
|
|
/// Less than operator used for sorting.
|
|
/// </summary>
|
|
/// <param name="other">The QTreeWidgetItem to compare against for sorting</param>
|
|
/// <returns>True if this is less than other, else false.</returns>
|
|
bool operator < (const QTreeWidgetItem& other) const override
|
|
{
|
|
const auto column = treeWidget()->sortColumn();
|
|
auto itemWidget1 = treeWidget()->itemWidget(const_cast<VariationTreeWidgetItem*>(this), 1);//Get the widget for the second column.
|
|
|
|
if (auto spinBox1 = dynamic_cast<VariationTreeDoubleSpinBox*>(itemWidget1))//Cast the widget to the VariationTreeDoubleSpinBox type.
|
|
{
|
|
auto itemWidget2 = treeWidget()->itemWidget(const_cast<QTreeWidgetItem*>(&other), 1);//Get the widget for the second column of the widget item passed in.
|
|
|
|
if (auto spinBox2 = dynamic_cast<VariationTreeDoubleSpinBox*>(itemWidget2))//Cast the widget to the VariationTreeDoubleSpinBox type.
|
|
{
|
|
if (spinBox1->IsParam() || spinBox2->IsParam())//Do not sort params, their order will always remain the same.
|
|
return false;
|
|
|
|
const auto weight1 = spinBox1->value();
|
|
const auto weight2 = spinBox2->value();
|
|
const auto index1 = spinBox1->GetVariationId();
|
|
const auto index2 = spinBox2->GetVariationId();
|
|
|
|
if (column == 0)//First column clicked, sort by variation index.
|
|
{
|
|
return index1 < index2;
|
|
}
|
|
else if (column == 1)//Second column clicked, sort by weight.
|
|
{
|
|
if (IsNearZero(weight1) && IsNearZero(weight2))
|
|
return index1 > index2;
|
|
else
|
|
return std::abs(weight1) < fabs(weight2);
|
|
}
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
eVariationId m_Id;
|
|
};
|