mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-07-11 18:54:53 -04:00
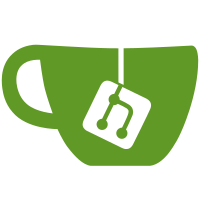
-Update various tooltips. -Increase precision of affine and xaos spinners. -Increase precision of fields written in Xml files to 8. --Bug fixes -When rendering on the CPU, if the number of threads didn't divide evenly into the number of rows, it would leave a blank spot on the last few rows. -Fix numerous parsing bugs when reading .chaos files. -Added compatibility fixes and/or optimizations to the following variations: asteria, bcircle, bcollide, bipolar, blob2, btransform, cell, circlecrop, circlecrop2, collideoscope, cpow2, cropn, cross, curl, depth_ngon2, depth_sine2, edisc, eRotate, escher, fan2, hex_rand, hypershift, hypershift2, hypertile1, julia, julian, julian2, juliaq, juliascope, lazyjess, log, loonie2, murl, murl2, npolar, oscilloscope2, perspective, phoenix_julia, sphericaln, squish, starblur, starblur2, truchet, truchet_glyph, waffle, wavesn.
79 lines
2.3 KiB
C++
79 lines
2.3 KiB
C++
#include "FractoriumPch.h"
|
|
#include "Fractorium.h"
|
|
#include <QtWidgets/QApplication>
|
|
#include <xmmintrin.h>
|
|
#include <immintrin.h>
|
|
#include <pmmintrin.h>
|
|
|
|
#ifdef __APPLE__
|
|
/// <summary>
|
|
/// Export default user data to ./config/fractorium
|
|
/// </summary>
|
|
void ExportUserData()
|
|
{
|
|
auto exec = new QProcess();
|
|
exec->setWorkingDirectory(QCoreApplication::applicationDirPath());
|
|
exec->start("/bin/sh", QStringList() << "fractorium-sh");
|
|
}
|
|
#endif
|
|
|
|
/// <summary>
|
|
/// Main program entry point for Fractorium.exe.
|
|
/// </summary>
|
|
/// <param name="argc">The number of command line arguments passed</param>
|
|
/// <param name="argv">The command line arguments passed</param>
|
|
/// <returns>0 if successful, else 1.</returns>
|
|
int main(int argc, char* argv[])
|
|
{
|
|
int rv = -1;
|
|
QApplication a(argc, argv);
|
|
#ifdef TEST_CL
|
|
QMessageBox::critical(nullptr, "Error", "Fractorium cannot be run in test mode, undefine TEST_CL first.");
|
|
return 1;
|
|
#endif
|
|
#ifdef ISAAC_FLAM3_DEBUG
|
|
QMessageBox::critical(nullptr, "Error", "Fractorium cannot be run in test mode, undefine ISAAC_FLAM3_DEBUG first.");
|
|
return 1;
|
|
#endif
|
|
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
|
|
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
|
|
auto vf = VarFuncs<float>::Instance();//Create instances that will stay alive until the program exits.
|
|
auto vlf = VariationList<float>::Instance();
|
|
auto palf = PaletteList<float>::Instance();
|
|
auto settings = FractoriumSettings::Instance();
|
|
#ifdef DO_DOUBLE
|
|
auto vd = VarFuncs<float>::Instance();
|
|
auto vld = VariationList<double>::Instance();//No further creations should occur after this.
|
|
#endif
|
|
|
|
try
|
|
{
|
|
//Required for large allocs, else GPU memory usage will be severely limited to small sizes.
|
|
//This must be done in the application and not in the EmberCL DLL.
|
|
#ifdef _WIN32
|
|
_putenv_s("GPU_MAX_ALLOC_PERCENT", "100");
|
|
#else
|
|
putenv(const_cast<char*>("GPU_MAX_ALLOC_PERCENT=100"));
|
|
#endif
|
|
Fractorium w;
|
|
w.show();
|
|
#ifdef __APPLE__
|
|
// exporting user data
|
|
ExportUserData();
|
|
#endif
|
|
a.installEventFilter(&w);
|
|
rv = a.exec();
|
|
}
|
|
catch (const std::exception& e)
|
|
{
|
|
QMessageBox::critical(nullptr, "Fatal Error", QString::fromStdString(e.what()));
|
|
}
|
|
catch (const char* e)
|
|
{
|
|
QMessageBox::critical(nullptr, "Fatal Error", e);
|
|
}
|
|
|
|
return rv;
|
|
}
|
|
|