mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-21 05:00:06 -05:00
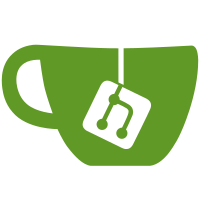
-Add new preset dimensions to the right click menu of the width and height fields in the editor. -Change QSS stylesheets to properly handle tabs. -Make tabs rectangular by default. For some reason, they had always been triangular. --Bug fixes -Incremental rendering times in the editor were wrong. --Code changes -Migrate to Qt6. There is probably more work to be done here. -Migrate to VS2022. -Migrate to Wix 4 installer. -Change installer to install to program files for all users. -Fix many VS2022 code analysis warnings. -No longer use byte typedef, because std::byte is now a type. Revert all back to unsigned char. -Upgrade OpenCL headers to version 3.0 and keep locally now rather than trying to look for system files. -No longer link to Nvidia or AMD specific OpenCL libraries. Use the generic installer located at OCL_ROOT too. -Add the ability to change OpenCL grid dimensions. This was attempted for investigating possible performance improvments, but made no difference. This has not been verified on Linux or Mac yet.
153 lines
5.2 KiB
C++
153 lines
5.2 KiB
C++
#include "FractoriumPch.h"
|
|
#include "Fractorium.h"
|
|
|
|
/// <summary>
|
|
/// Initialize the xforms selection UI.
|
|
/// </summary>
|
|
void Fractorium::InitXformsSelectUI()
|
|
{
|
|
m_XformsSelectionLayout = dynamic_cast<QFormLayout*>(ui.XformsSelectGroupBoxScrollAreaWidget->layout());
|
|
connect(ui.XformsSelectAllButton, SIGNAL(clicked(bool)), this, SLOT(OnXformsSelectAllButtonClicked(bool)), Qt::QueuedConnection);
|
|
connect(ui.XformsSelectNoneButton, SIGNAL(clicked(bool)), this, SLOT(OnXformsSelectNoneButtonClicked(bool)), Qt::QueuedConnection);
|
|
ClearXformsSelections();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Check all of the xform selection checkboxes.
|
|
/// </summary>
|
|
/// <param name="checked">Ignored</param>
|
|
void Fractorium::OnXformsSelectAllButtonClicked(bool checked) { ForEachXformCheckbox([&](int i, QCheckBox * w, bool isFinal) { w->setChecked(true); }); }
|
|
|
|
/// <summary>
|
|
/// Uncheck all of the xform selection checkboxes.
|
|
/// </summary>
|
|
/// <param name="checked">Ignored</param>
|
|
void Fractorium::OnXformsSelectNoneButtonClicked(bool checked) { ForEachXformCheckbox([&](int i, QCheckBox * w, bool isFinal) { w->setChecked(false); }); }
|
|
|
|
/// <summary>
|
|
/// Return whether the checkbox at the specified index is checked.
|
|
/// </summary>
|
|
/// <param name="i">The index of the xform to check for selection</param>
|
|
/// <param name="checked">True if checked, else false.</param>
|
|
bool Fractorium::IsXformSelected(size_t i)
|
|
{
|
|
if (i < m_XformSelections.size())
|
|
if (const auto w = m_XformSelections[i])
|
|
return w->isChecked();
|
|
|
|
return false;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Return the number of xforms selected, optionally counting the final xform.
|
|
/// </summary>
|
|
/// <param name="includeFinal">Whether to include the final in the count if selected.</param>
|
|
/// <returns>The caption string</returns>
|
|
int Fractorium::SelectedXformCount(bool includeFinal)
|
|
{
|
|
auto selCount = 0;
|
|
ForEachXformCheckbox([&](int index, QCheckBox * cb, bool isFinal)
|
|
{
|
|
if (cb->isChecked())
|
|
{
|
|
if (!isFinal || includeFinal)
|
|
selCount++;
|
|
}
|
|
});
|
|
return selCount;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Clear all of the dynamically created xform checkboxes.
|
|
/// </summary>
|
|
void Fractorium::ClearXformsSelections()
|
|
{
|
|
QLayoutItem* child = nullptr;
|
|
m_XformSelections.clear();
|
|
m_XformsSelectionLayout->blockSignals(true);
|
|
|
|
while (m_XformsSelectionLayout->count() && (child = m_XformsSelectionLayout->takeAt(0)))
|
|
{
|
|
auto w = child->widget();
|
|
delete child;
|
|
delete w;
|
|
}
|
|
|
|
m_XformsSelectionLayout->blockSignals(false);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Make a caption from an xform.
|
|
/// The caption will be the xform count + 1, optionally followed by the xform's name.
|
|
/// For final xforms, the string "Final" will be used in place of the count.
|
|
/// </summary>
|
|
/// <param name="i">The index of the xform to make a caption for</param>
|
|
/// <returns>The caption string</returns>
|
|
template <typename T>
|
|
QString FractoriumEmberController<T>::MakeXformCaption(size_t i)
|
|
{
|
|
const auto forceFinal = m_Fractorium->HaveFinal();
|
|
const auto isFinal = m_Ember.FinalXform() == m_Ember.GetTotalXform(i, forceFinal);
|
|
QString caption = isFinal ? "Final" : QString::number(i + 1);
|
|
|
|
if (const auto xform = m_Ember.GetTotalXform(i, forceFinal))
|
|
if (!xform->m_Name.empty())
|
|
caption += " (" + QString::fromStdString(xform->m_Name) + ")";
|
|
|
|
return caption;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Function to perform the specified operation on every dynamically created xform selection checkbox.
|
|
/// </summary>
|
|
/// <param name="func">The operation to perform which is a function taking the index of the xform, its checkbox, and a bool specifying whether it's final.</param>
|
|
void Fractorium::ForEachXformCheckbox(std::function<void(int, QCheckBox*, bool)> func)
|
|
{
|
|
int i = 0;
|
|
const auto haveFinal = HaveFinal();
|
|
|
|
for (auto& cb : m_XformSelections)
|
|
func(i++, cb, haveFinal && cb == m_XformSelections.back());
|
|
}
|
|
|
|
/// <summary>
|
|
/// Function to perform the specified operation on one dynamically created xform selection checkbox.
|
|
/// </summary>
|
|
/// <param name="i">The index of the checkbox</param>
|
|
/// <param name="func">The operation to perform</param>
|
|
/// <returns>True if the checkbox was found, else false.</returns>
|
|
template <typename T>
|
|
bool FractoriumEmberController<T>::XformCheckboxAt(int i, std::function<void(QCheckBox*)> func)
|
|
{
|
|
if (i < m_Fractorium->m_XformSelections.size())
|
|
{
|
|
if (const auto w = m_Fractorium->m_XformSelections[i])
|
|
{
|
|
func(w);
|
|
return true;
|
|
}
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Function to perform the specified operation on one dynamically created xform selection checkbox.
|
|
/// The checkbox is specified by the xform it corresponds to, rather than its index.
|
|
/// </summary>
|
|
/// <param name="xform">The xform that corresponds to the checkbox</param>
|
|
/// <param name="func">The operation to perform</param>
|
|
/// <returns>True if the checkbox was found, else false.</returns>
|
|
template <typename T>
|
|
bool FractoriumEmberController<T>::XformCheckboxAt(Xform<T>* xform, std::function<void(QCheckBox*)> func)
|
|
{
|
|
const auto forceFinal = m_Fractorium->HaveFinal();
|
|
return XformCheckboxAt(m_Ember.GetTotalXformIndex(xform, forceFinal), func);
|
|
}
|
|
|
|
template class FractoriumEmberController<float>;
|
|
|
|
#ifdef DO_DOUBLE
|
|
template class FractoriumEmberController<double>;
|
|
#endif
|