mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-01-21 05:00:06 -05:00
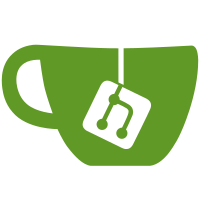
-Show image index in the library and sequence trees. -Add progress bar showing when preview renders are complete. -Automatically show animation preview when sequence generation is done. -Add the ability in the final render dialog to start a render within a sequence from a given point, rather than always having to start from the beginning. --Allow using the image index as its name rather than the file name.
364 lines
9.2 KiB
C++
364 lines
9.2 KiB
C++
#pragma once
|
|
|
|
#include "FractoriumPch.h"
|
|
|
|
/// <summary>
|
|
/// FractoriumSettings class.
|
|
/// </summary>
|
|
|
|
#define EARLYCLIP "render/earlyclip"
|
|
#define YAXISUP "render/yaxisup"
|
|
#define TRANSPARENCY "render/transparency"
|
|
#define OPENCL "render/opencl"
|
|
#define SHAREDTEXTURE "render/sharedtexture"
|
|
#define DOUBLEPRECISION "render/dp64"
|
|
#define CONTUPDATE "render/continuousupdate"
|
|
#define SHOWALLXFORMS "render/dragshowallxforms"
|
|
#define SHOWXFORMS "render/showxforms"
|
|
#define SHOWGRID "render/showgrid"
|
|
#define TOGGLETYPE "render/toggletype"
|
|
#define PNG16BIT "render/png16bit"
|
|
#define DEVICES "render/devices"
|
|
#define THREADCOUNT "render/threadcount"
|
|
#define CPUDEFILTER "render/cpudefilter"
|
|
#define OPENCLDEFILTER "render/opencldefilter"
|
|
#define CPUSUBBATCH "render/cpusubbatch"
|
|
#define OPENCLSUBBATCH "render/openclsubbatch"
|
|
#define OPENCLSUBBATCHPCT "render/openclsubbatchpct"
|
|
#define RANDOMCOUNT "render/randomcount"
|
|
#define CPUQUALITY "render/cpuquality"
|
|
#define OPENCLQUALITY "render/openclquality"
|
|
#define LOADLAST "render/loadlastonstart"
|
|
#define ROTSCALE "render/rotateandscale"
|
|
#define FLAM3COMPAT "render/flam3compat"
|
|
|
|
#define STAGGER "sequence/stagger"
|
|
#define STAGGERMAX "sequence/staggermax"
|
|
#define FRAMESPERROT "sequence/framesperrot"
|
|
#define FRAMESPERROTMAX "sequence/framesperrotmax"
|
|
#define ROTATIONS "sequence/rotations"
|
|
#define ROTATIONSMAX "sequence/rotationsmax"
|
|
#define BLENDFRAMES "sequence/blendframes"
|
|
#define BLENDFRAMESMAX "sequence/blendframesmax"
|
|
#define ROTATIONSPERBLEND "sequence/rotationsperblend"
|
|
#define ROTATIONSPERBLENDMAX "sequence/rotationsperblendmax"
|
|
#define LINEAR "sequence/linear"
|
|
#define ANIMATION_FPS "sequence/animationfps"
|
|
|
|
#define VARFILTERSUM "varfilter/sumcheckbox"
|
|
#define VARFILTERASSIGN "varfilter/assigncheckbox"
|
|
#define VARFILTERPPSUM "varfilter/ppsumcheckbox"
|
|
#define VARFILTERPPASSIGN "varfilter/ppassigncheckbox"
|
|
#define VARFILTERSDC "varfilter/dccheckbox"
|
|
#define VARFILTERSTATE "varfilter/statecheckbox"
|
|
#define VARFILTERPARAM "varfilter/paramcheckbox"
|
|
#define VARFILTERNONPARAM "varfilter/nonparamcheckbox"
|
|
|
|
#define FINALEARLYCLIP "finalrender/earlyclip"
|
|
#define FINALYAXISUP "finalrender/finalyaxisup"
|
|
#define FINALTRANSPARENCY "finalrender/transparency"
|
|
#define FINALOPENCL "finalrender/opencl"
|
|
#define FINALDOUBLEPRECISION "finalrender/dp64"
|
|
#define FINALSAVEXML "finalrender/savexml"
|
|
#define FINALDOALL "finalrender/doall"
|
|
#define FINALDOSEQUENCE "finalrender/dosequence"
|
|
#define FINALPNG16BIT "finalrender/png16bit"
|
|
#define FINALKEEPASPECT "finalrender/keepaspect"
|
|
#define FINALSCALE "finalrender/scale"
|
|
#define FINALEXT "finalrender/ext"
|
|
#define FINALDEVICES "finalrender/devices"
|
|
#define FINALTHREADCOUNT "finalrender/threadcount"
|
|
#define FINALTHREADPRIORITY "finalrender/threadpriority"
|
|
#define FINALOPENCLSUBBATCHPCT "finalrender/openclsubbatchpct"
|
|
#define FINALQUALITY "finalrender/quality"
|
|
#define FINALTEMPORALSAMPLES "finalrender/temporalsamples"
|
|
#define FINALSUPERSAMPLE "finalrender/supersample"
|
|
#define FINALSTRIPS "finalrender/strips"
|
|
#define FINALUSENUMBERS "finalrender/usenumbers"
|
|
|
|
#define XMLWIDTH "xml/width"
|
|
#define XMLHEIGHT "xml/height"
|
|
#define XMLTEMPORALSAMPLES "xml/temporalsamples"
|
|
#define XMLQUALITY "xml/quality"
|
|
#define XMLSUPERSAMPLE "xml/supersample"
|
|
|
|
#define OPENFOLDER "path/open"
|
|
#define OPENPALETTEIMAGEFOLDER "path/openpaletteimage"
|
|
#define SAVEFOLDER "path/save"
|
|
|
|
#define OPENXMLEXT "file/openxmlext"
|
|
#define SAVEXMLEXT "file/savexmlext"
|
|
#define OPENIMAGEEXT "file/openimageext"
|
|
#define SAVEIMAGEEXT "file/saveimageext"
|
|
#define AUTOUNIQUE "file/autounique"
|
|
|
|
#define IDENTITYID "identity/id"
|
|
#define IDENTITYURL "identity/url"
|
|
#define IDENTITYNICK "identity/nick"
|
|
|
|
#define UIVARIATIONS "ui/variations"
|
|
|
|
#define STYLETHEME "style/theme"
|
|
|
|
/// <summary>
|
|
/// Class for preserving various program options between
|
|
/// runs of Fractorium. Each of these generally corresponds
|
|
/// to items in the options dialog and the final render dialog.
|
|
/// </summary>
|
|
class FractoriumSettings : public QSettings, public Singleton<FractoriumSettings>
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
SINGLETON_DERIVED_IMPL(FractoriumSettings);
|
|
void EnsureDefaults();
|
|
|
|
bool EarlyClip();
|
|
void EarlyClip(bool b);
|
|
|
|
bool YAxisUp();
|
|
void YAxisUp(bool b);
|
|
|
|
bool Transparency();
|
|
void Transparency(bool b);
|
|
|
|
bool OpenCL();
|
|
void OpenCL(bool b);
|
|
|
|
bool SharedTexture();
|
|
void SharedTexture(bool b);
|
|
|
|
bool Double();
|
|
void Double(bool b);
|
|
|
|
bool ShowAllXforms();
|
|
void ShowAllXforms(bool b);
|
|
|
|
bool ShowXforms();
|
|
void ShowXforms(bool b);
|
|
|
|
bool ShowGrid();
|
|
void ShowGrid(bool b);
|
|
|
|
bool ToggleType();
|
|
void ToggleType(bool b);
|
|
|
|
bool Png16Bit();
|
|
void Png16Bit(bool b);
|
|
|
|
bool ContinuousUpdate();
|
|
void ContinuousUpdate(bool b);
|
|
|
|
QList<QVariant> Devices();
|
|
void Devices(const QList<QVariant>& devices);
|
|
|
|
uint ThreadCount();
|
|
void ThreadCount(uint i);
|
|
|
|
bool CpuDEFilter();
|
|
void CpuDEFilter(bool b);
|
|
|
|
bool OpenCLDEFilter();
|
|
void OpenCLDEFilter(bool b);
|
|
|
|
uint CpuSubBatch();
|
|
void CpuSubBatch(uint i);
|
|
|
|
double OpenCLSubBatchPct();
|
|
void OpenCLSubBatchPct(double d);
|
|
|
|
uint OpenCLSubBatch();
|
|
void OpenCLSubBatch(uint i);
|
|
|
|
uint RandomCount();
|
|
void RandomCount(uint i);
|
|
|
|
uint CpuQuality();
|
|
void CpuQuality(uint i);
|
|
|
|
uint OpenClQuality();
|
|
void OpenClQuality(uint i);
|
|
|
|
bool LoadLast();
|
|
void LoadLast(bool b);
|
|
|
|
bool RotateAndScale();
|
|
void RotateAndScale(bool b);
|
|
|
|
bool Flam3Compat();
|
|
void Flam3Compat(bool b);
|
|
|
|
double Stagger();
|
|
void Stagger(double i);
|
|
|
|
double StaggerMax();
|
|
void StaggerMax(double i);
|
|
|
|
uint FramesPerRot();
|
|
void FramesPerRot(uint i);
|
|
|
|
uint FramesPerRotMax();
|
|
void FramesPerRotMax(uint i);
|
|
|
|
uint Rotations();
|
|
void Rotations(double d);
|
|
|
|
uint RotationsMax();
|
|
void RotationsMax(double d);
|
|
|
|
uint BlendFrames();
|
|
void BlendFrames(uint i);
|
|
|
|
uint BlendFramesMax();
|
|
void BlendFramesMax(uint i);
|
|
|
|
uint RotationsPerBlend();
|
|
void RotationsPerBlend(uint i);
|
|
|
|
uint RotationsPerBlendMax();
|
|
void RotationsPerBlendMax(uint i);
|
|
|
|
bool Linear();
|
|
void Linear(bool b);
|
|
|
|
uint AnimationFps();
|
|
void AnimationFps(uint i);
|
|
|
|
int VarFilterSum();
|
|
void VarFilterSum(int i);
|
|
|
|
int VarFilterAssign();
|
|
void VarFilterAssign(int i);
|
|
|
|
int VarFilterPpsum();
|
|
void VarFilterPpsum(int i);
|
|
|
|
int VarFilterPpassign();
|
|
void VarFilterPpassign(int i);
|
|
|
|
int VarFilterSdc();
|
|
void VarFilterSdc(int i);
|
|
|
|
int VarFilterState();
|
|
void VarFilterState(int i);
|
|
|
|
int VarFilterParam();
|
|
void VarFilterParam(int i);
|
|
|
|
int VarFilterNonparam();
|
|
void VarFilterNonparam(int i);
|
|
|
|
bool FinalEarlyClip();
|
|
void FinalEarlyClip(bool b);
|
|
|
|
bool FinalYAxisUp();
|
|
void FinalYAxisUp(bool b);
|
|
|
|
bool FinalTransparency();
|
|
void FinalTransparency(bool b);
|
|
|
|
bool FinalOpenCL();
|
|
void FinalOpenCL(bool b);
|
|
|
|
bool FinalDouble();
|
|
void FinalDouble(bool b);
|
|
|
|
bool FinalSaveXml();
|
|
void FinalSaveXml(bool b);
|
|
|
|
bool FinalDoAll();
|
|
void FinalDoAll(bool b);
|
|
|
|
bool FinalDoSequence();
|
|
void FinalDoSequence(bool b);
|
|
|
|
bool FinalUseNumbers();
|
|
void FinalUseNumbers(bool b);
|
|
|
|
bool FinalPng16Bit();
|
|
void FinalPng16Bit(bool b);
|
|
|
|
bool FinalKeepAspect();
|
|
void FinalKeepAspect(bool b);
|
|
|
|
uint FinalScale();
|
|
void FinalScale(uint i);
|
|
|
|
QString FinalExt();
|
|
void FinalExt(const QString& s);
|
|
|
|
QList<QVariant> FinalDevices();
|
|
void FinalDevices(const QList<QVariant>& devices);
|
|
|
|
uint FinalThreadCount();
|
|
void FinalThreadCount(uint i);
|
|
|
|
int FinalThreadPriority();
|
|
void FinalThreadPriority(int b);
|
|
|
|
double FinalOpenCLSubBatchPct();
|
|
void FinalOpenCLSubBatchPct(double d);
|
|
|
|
uint FinalQuality();
|
|
void FinalQuality(uint i);
|
|
|
|
uint FinalTemporalSamples();
|
|
void FinalTemporalSamples(uint i);
|
|
|
|
uint FinalSupersample();
|
|
void FinalSupersample(uint i);
|
|
|
|
size_t FinalStrips();
|
|
void FinalStrips(size_t i);
|
|
|
|
uint XmlTemporalSamples();
|
|
void XmlTemporalSamples(uint i);
|
|
|
|
uint XmlQuality();
|
|
void XmlQuality(uint i);
|
|
|
|
uint XmlSupersample();
|
|
void XmlSupersample(uint i);
|
|
|
|
QString OpenFolder();
|
|
void OpenFolder(const QString& s);
|
|
|
|
QString OpenPaletteImageFolder();
|
|
void OpenPaletteImageFolder(const QString& s);
|
|
|
|
QString SaveFolder();
|
|
void SaveFolder(const QString& s);
|
|
|
|
QString OpenXmlExt();
|
|
void OpenXmlExt(const QString& s);
|
|
|
|
QString SaveXmlExt();
|
|
void SaveXmlExt(const QString& s);
|
|
|
|
QString OpenImageExt();
|
|
void OpenImageExt(const QString& s);
|
|
|
|
QString SaveImageExt();
|
|
void SaveImageExt(const QString& s);
|
|
|
|
bool SaveAutoUnique();
|
|
void SaveAutoUnique(bool b);
|
|
|
|
QString Id();
|
|
void Id(const QString& s);
|
|
|
|
QString Url();
|
|
void Url(const QString& s);
|
|
|
|
QString Nick();
|
|
void Nick(const QString& s);
|
|
|
|
QMap<QString, QVariant> Variations();
|
|
void Variations(const QMap<QString, QVariant>& m);
|
|
|
|
QString Theme();
|
|
void Theme(const QString& s);
|
|
|
|
private:
|
|
FractoriumSettings();
|
|
};
|