mirror of
https://bitbucket.org/mfeemster/fractorium.git
synced 2025-06-30 13:26:02 -04:00
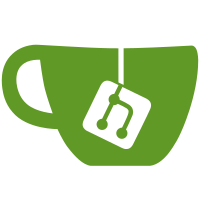
-Add new preset dimensions to the right click menu of the width and height fields in the editor. -Change QSS stylesheets to properly handle tabs. -Make tabs rectangular by default. For some reason, they had always been triangular. --Bug fixes -Incremental rendering times in the editor were wrong. --Code changes -Migrate to Qt6. There is probably more work to be done here. -Migrate to VS2022. -Migrate to Wix 4 installer. -Change installer to install to program files for all users. -Fix many VS2022 code analysis warnings. -No longer use byte typedef, because std::byte is now a type. Revert all back to unsigned char. -Upgrade OpenCL headers to version 3.0 and keep locally now rather than trying to look for system files. -No longer link to Nvidia or AMD specific OpenCL libraries. Use the generic installer located at OCL_ROOT too. -Add the ability to change OpenCL grid dimensions. This was attempted for investigating possible performance improvments, but made no difference. This has not been verified on Linux or Mac yet.
146 lines
4.0 KiB
C++
146 lines
4.0 KiB
C++
#pragma once
|
|
|
|
#include "EmberDefines.h"
|
|
|
|
namespace EmberNs
|
|
{
|
|
/// <summary>
|
|
/// Thin derivation of pair<eEmberMotionParam, T> for the element type
|
|
/// of EmberMotion<T>::m_MotionParams to allow for copying vectors
|
|
/// of different types of T.
|
|
/// Template argument expected to be float or double.
|
|
/// </summary>
|
|
template <typename T>
|
|
class EMBER_API MotionParam : public pair <eEmberMotionParam, T>
|
|
{
|
|
public:
|
|
/// <summary>
|
|
/// Default constructor, which calls the base, which sets first and second to their defaults.
|
|
/// </summary>
|
|
MotionParam() = default;
|
|
|
|
/// <summary>
|
|
/// Member-wise constructor.
|
|
/// </summary>
|
|
/// <param name="e">The eEmberMotionParam value to assign to first</param>
|
|
/// <param name="t">The T value to assign to second</param>
|
|
MotionParam(eEmberMotionParam e, T t)
|
|
: pair<eEmberMotionParam, T>(e, t)
|
|
{
|
|
}
|
|
|
|
/// <summary>
|
|
/// Default copy constructor.
|
|
/// </summary>
|
|
/// <param name="other">The MotionParam object to copy</param>
|
|
MotionParam(const MotionParam<T>& other)
|
|
: pair <eEmberMotionParam, T>()
|
|
{
|
|
operator=<T>(other);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Copy constructor to copy a MotionParam object of type U.
|
|
/// </summary>
|
|
/// <param name="other">The MotionParam object to copy</param>
|
|
template <typename U>
|
|
MotionParam(const MotionParam<U>& other)
|
|
{
|
|
operator=<U>(other);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Default assignment operator.
|
|
/// </summary>
|
|
/// <param name="other">The MotionParam object to copy</param>
|
|
MotionParam<T>& operator = (const MotionParam<T>& other)
|
|
{
|
|
if (this != &other)
|
|
MotionParam<T>::operator=<T>(other);
|
|
|
|
return *this;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Assignment operator to assign a MotionParam object of type U.
|
|
/// </summary>
|
|
/// <param name="other">The MotionParam object to copy.</param>
|
|
/// <returns>Reference to updated self</returns>
|
|
template <typename U>
|
|
MotionParam& operator = (const MotionParam<U>& other)
|
|
{
|
|
this->first = other.first;
|
|
this->second = static_cast<T>(other.second);
|
|
return *this;
|
|
}
|
|
};
|
|
|
|
/// <summary>
|
|
/// EmberMotion elements allow for motion of the flame parameters such as zoom, yaw, pitch and friends
|
|
/// The values in these elements can be used to modify flame parameters during rotation in much the same
|
|
/// way as motion elements on xforms do.
|
|
/// Template argument expected to be float or double.
|
|
/// </summary>
|
|
template <typename T>
|
|
class EMBER_API EmberMotion
|
|
{
|
|
public:
|
|
/// <summary>
|
|
/// Default constructor to initialize motion freq and offset to 0 and the motion func to SIN.
|
|
/// </summary>
|
|
EmberMotion() = default;
|
|
~EmberMotion() = default;
|
|
|
|
/// <summary>
|
|
/// Default copy constructor.
|
|
/// </summary>
|
|
/// <param name="other">The EmberMotion object to copy</param>
|
|
EmberMotion(const EmberMotion<T>& other)
|
|
{
|
|
operator=<T>(other);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Copy constructor to copy a EmberMotion object of type U.
|
|
/// </summary>
|
|
/// <param name="other">The EmberMotion object to copy</param>
|
|
template <typename U>
|
|
EmberMotion(const EmberMotion<U>& other)
|
|
{
|
|
operator=<U>(other);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Default assignment operator.
|
|
/// </summary>
|
|
/// <param name="other">The EmberMotion object to copy</param>
|
|
EmberMotion<T>& operator = (const EmberMotion<T>& other)
|
|
{
|
|
if (this != &other)
|
|
EmberMotion<T>::operator=<T>(other);
|
|
|
|
return *this;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Assignment operator to assign a EmberMotion object of type U.
|
|
/// </summary>
|
|
/// <param name="other">The EmberMotion object to copy.</param>
|
|
/// <returns>Reference to updated self</returns>
|
|
template <typename U>
|
|
EmberMotion& operator = (const EmberMotion<U>& other)
|
|
{
|
|
CopyCont(m_MotionParams, other.m_MotionParams);
|
|
m_MotionFunc = other.m_MotionFunc;
|
|
m_MotionFreq = static_cast<T>(other.m_MotionFreq);
|
|
m_MotionOffset = static_cast<T>(other.m_MotionOffset);
|
|
return *this;
|
|
}
|
|
|
|
T m_MotionFreq = 0;
|
|
T m_MotionOffset = 0;
|
|
eMotion m_MotionFunc = eMotion::MOTION_SIN;
|
|
vector<MotionParam<T>> m_MotionParams;
|
|
};
|
|
}
|